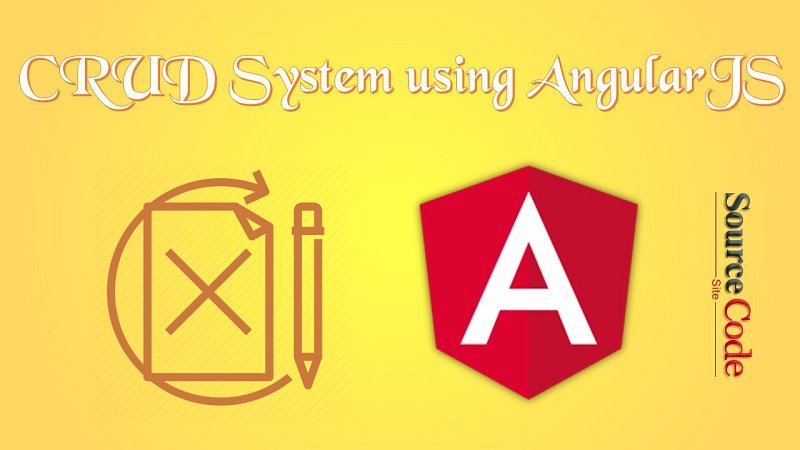
CRUD System using AngularJS
Previous Angular JS arcitcle we have learned How To Create CRUD System in PHP using Angular js, Today we will Learn How to create Simple CRUD System using AngularJS. in this article we are focus on creating, reading, updating, and deleting records. we will do it using Angular JS. if you are a senior web developer you must have created plenty of CRUD system already. They maybe exist in a content management system, and inventory management system OR accounting application. if you just started web development, you are certainly going to experience lots CRUD grid’s creation work in your later career. The main purpose of a CRUD system is that enables users create, read, update, and delete data.
CRUD Operations
C – Create OR Insert data to MySQL Database.
R – Read Database Records.
U – Update Selected MySQL Records
D – Delete Selected Record From MySQL Database.
1 – Creating a Form
in this form i just added a modal for add data, update data and delete data dynamically.In this below source code I just add some function to make the update and delete data.
ng-click="selectuser(user)"
will store the value of the indexing array in the table when clicked by the user. ng-click="deleteuser"
will store the index of the targeted user data when clicked.
<!DOCTYPE html> <html lang = "en"> <head> <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/> <title>Sourcecodesite.com - CRUD System using AngularJS</title> <link rel = "stylesheet" type = "text/css" href = "css/bootstrap.css" /> <script src = "js/angular.js"></script> <script src = "js/app.js"></script> <style> .card { box-shadow: 0 10px 20px rgba(0,0,0,0.19), 0 6px 6px rgba(0,0,0,0.23); } </style> </head> <body ng-app="myModule" ng-controller="myController" style="margin:55px; background-color:rgba(255,155,180,210);"> <div class="row"> <div class="col-md-2"></div> <div class="col-md-8 well card"> <h3 class="text-danger"><marquee behavior="alternate" OnMouseOver="stop();" OnMouseOut="start();">CRUD System using AngularJS</marquee></h3> <hr class="card" style="border-top:1px solid #000;"/> <div class="alert alert-success">users Personal Information <button class="btn btn-sm btn-success pull-right" data-toggle="modal" data-target="#add_user"> <span class="glyphicon glyphicon-plus"> </span> </button> </div> <div class="container-fluid"> <br /> <br /> <table class="table table-bordered alert-success"> <thead> <tr> <th>ID</th> <th>Full Name</th> <th>Email</th> <th>Mobile</th> <th>Country</th> <th colspan="2">Action</th> </tr> </thead> <tbody> <tr ng-repeat = "user in users"> <td>{{$index+1}}</td> <td>{{user.name}}</td> <td>{{user.email}}</td> <td>{{user.mobile}}</td> <td>{{user.country}}</td> <td><button type="button" data-toggle="modal" data-target="#update_user" ng-click = "selectuser(user)" class="btn btn-sm btn-warning"><span class="glyphicon glyphicon-edit"></span> Update</button></td> <td><button type="button" data-toggle = "modal" data-target = "#delete_user" class="btn btn-sm btn-danger"><span class="glyphicon glyphicon-trash"></span> Delete</button></td> </tr> </tbody> </table> </div> <div class="alert alert-primary text-center">SourceCode Design By <a href="http://sourcecodessite.com" target="_blank">SourceCodesite.com</a> </div> </div> </div> <div class="modal fade" id="add_user" tabindex="-1" role="dialog" aria-labelledby="myModalLabel"> <div class="modal-dialog" role="document"> <div class="modal-content"> <form> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button> <h4 class="modal-title text-info" id="myModalLabel">Add User</h4> </div> <div class="modal-body"> <div class="form-group"> <label>Full Name</label> <input type="text" class="form-control" ng-model="newuser.name"/> </div> <div class="form-group"> <label>Email</label> <input type="email" class="form-control" ng-model="newuser.email"/> </div> <div class="form-group"> <label>Mobile No.</label> <input type="text" class="form-control" ng-model="newuser.mobile"/> </div> <div class="form-group"> <label>Country</label> <select class="form-control" ng-model="newuser.country" > <option value="">Choose an option</option> <option ng-repeat="country in countries" value="{{country.code}}">{{country.name}}</option> </select> </div> </div> <div class="modal-footer"> <button class="btn btn-primary" ng-click="saveuser()" data-dismiss="modal"><span class="glyphicon glyphicon-save"></span> Save</button> </div> </form> </div> </div> </div> <div class="modal fade" id="update_user" tabindex="-1" role="dialog" aria-labelledby="myModalLabel"> <div class="modal-dialog" role="document"> <div class="modal-content"> <form> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button> <h4 class="modal-title text-info" id="myModalLabel">Update User</h4> </div> <div class="modal-body"> <div class = "form-group"> <label>Full Name</label> <input type="text" class="form-control" ng-model="selecteduser.name"/> </div> <div class="form-group"> <label>Email</label> <input type="email" class="form-control" ng-model="selecteduser.email"/> </div> <div class="form-group"> <label>Mobile</label> <input type="text" class="form-control" ng-model="selecteduser.mobile"/> </div> <div class="form-group"> <label>Country</label> <select class="form-control" ng-model="selecteduser.country" > <option value="">Choose an option</option> <option ng-repeat="country in countries" value="{{country.code}}">{{country.name}}</option> </select> </select> </div> </div> <div class="modal-footer"> <button class="btn btn-success" data-dismiss="modal" ng-click="update_user()"><span class="glyphicon glyphicon-edit"></span> Update</button> </div> </form> </div> </div> </div> <div class="modal fade" id="delete_user" tabindex="-1" role="dialog" aria-labelledby="myModalLabel"> <div class="modal-dialog" role="document"> <div class="modal-content"> <form> <div class="modal-body"> <center><h4 class="text-danger">Are you sure you want to delete this record?</h4></center> </div> <div class="modal-footer"> <button class="btn btn-danger" data-dismiss="modal" ng-click="deleteuser()"><span class="glyphicon glyphicon-check"></span> Yes</button> <button class="btn btn-success" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> No</button> </div> </form> </div> </div> </div> </body> <!-- _______________________________________________ | Source Code Created By : Sourcecodesite.com | | Author : Priyanshu Raj | | Website : http://sourcecodessite.com | |______________________________________________| --> <script src="js/jquery-3.1.1.js"></script> <script src="js/bootstrap.js"></script> </html>
2 – Create Function using AngularJS To Controls CRUD Operations
in this step we are creating function using AngularJs Directives to performs CRUD Operations. Source Code Given Below.
var app = angular.module("myModule", []) .controller("myController", function($scope){ $scope.newuser = {}; $scope.clickedusers = []; $scope.users = [ {name: "Raj", email: "example@mail.com", mobile: "8545005272", country: "IN"}, {name: "Abhinandan", email: "example@mail.com", mobile: "8545005272", country: "PK"}, {name: "Jagriti", email: "example@mail.com", mobile: "8545005272", country: "DZ"}, {name: "Priyanshu", email: "example@mail.com", mobile: "8545005272", country: "AS"}, ]; $scope.saveuser = function(){ $scope.users.push($scope.newuser); $scope.newuser = {}; }; $scope.selectuser = function(user){ $scope.selecteduser = user; }; $scope.updateuser = function(){ }; $scope.deleteuser = function(){ $scope.users.splice($scope.users.indexOf($scope.selectuser), 1); }; $scope.countries = [ {name: 'Afghanistan', code: 'AF'}, {name: 'Åland Islands', code: 'AX'}, {name: 'Albania', code: 'AL'}, {name: 'Algeria', code: 'DZ'}, {name: 'India', code: 'IN'}, {name: 'Pakistan', code: 'PK'}, {name: 'American Samoa', code: 'AS'} ]; }); /* _______________________________________________ | Source Code Created By : Sourcecodesite.com | | Author : Deepak Raj | | Website : http://sourcecodessite.com | |______________________________________________| */
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.