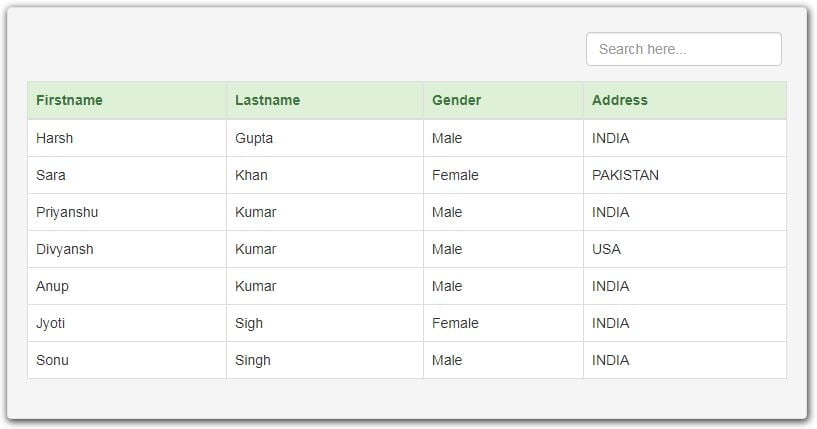
How To Create Search Filter in AngularJS
Hi Freinds, in this article we are going to learn How To Create Search Filter in AngularJS. We use the Search filter to get data easily by First Name, Last Name, Gender, and Address. As we type in the search box, all the columns in the table must be searched and only the matching rows should be displayed.
Getting Bootstrap
Bootstrap can be Download to your needs from their Getting Started page but I would prefer using the CDN option, because it is faster also it is advised to go through and get yourself accustomed with some bootstrap terms, including common classes. This page also some Examples of how to use Bootstrap classes.
Required Libraries
in this source code, we are using AangularJS main library file you can use their CDN by following these links.
1 – Creating Index Page
in this step, we are going to create an index page where show all data to perform search filter. here the source code you can save it as index.html.
<!DOCTYPE html> <html lang="en" ng-app="myModule"> <head> <title>How To Create Search Filter in AngularJS</title> <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1" /> <link rel="stylesheet" type="text/css" href="css/bootstrap.css" /> <style type="text/css"> body { margin-top: 150px; } .well { -webkit-box-shadow: 1px 2px 11px 1px rgba(0, 0, 0, 0.75); -moz-box-shadow: 1px 2px 11px 1px rgba(0, 0, 0, 0.75); box-shadow: 1px 2px 11px 1px rgba(0, 0, 0, 0.75); } .form-inline { float: right; padding: 5px; margin-bottom: 10px; } </style> </head> <body ng-controller="myController"> <div class="col-md-3"></div> <div class="col-md-6 well"> <div class="form-inline"> <input type="text" ng-model="filter" class="form-control" placeholder="Search here..." /> </div> <br /> <table class="table table-bordered"> <thead class="alert-success"> <tr> <th>Firstname</th> <th>Lastname</th> <th>Gender</th> <th>Address</th> </tr> </thead> <tbody style="background-color:#fff;"> <tr ng-repeat="member in members | filter: search | orderBy: 'lastname'"> <td>{{member.firstname}}</td> <td>{{member.lastname}}</td> <td>{{member.gender}}</td> <td>{{member.address}}</td> </tr> </tbody> </table> </div> <script src="js/angular.js"> < script src = "js/script.js" > < /body> </html >
2 – Creating Script For Search Filter
in this step we are going to create script in angular js for the search filter. here the source code you can save it as script.js.
var app = angular.module("myModule", []).controller("myController", function ($scope) { var members = [{ firstname: "Priyanshu", lastname: "Kumar", gender: "Male", address: "INDIA" }, { firstname: "Jyoti", lastname: "Sigh", gender: "Female", address: "INDIA" }, { firstname: "Divyansh", lastname: "Kumar", gender: "Male", address: "USA" }, { firstname: "Harsh", lastname: "Gupta", gender: "Male", address: "INDIA" }, { firstname: "Sara", lastname: "Khan", gender: "Female", address: "PAKISTAN" }, { firstname: "Anup", lastname: "Kumar", gender: "Male", address: "INDIA" }, { firstname: "Sonu", lastname: "Singh", gender: "Male", address: "INDIA" }]; $scope.members = members; $scope.search = function (keyword) { if ($scope.filter == undefined) { return true; } else { if (keyword.firstname.toLowerCase().indexOf($scope.filter.toLowerCase()) != -1 || keyword.lastname.toLowerCase().indexOf($scope.filter.toLowerCase()) != -1 || keyword.address.toLowerCase().indexOf($scope.filter.toLowerCase()) != -1) { return true; } } return false; } }); If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.