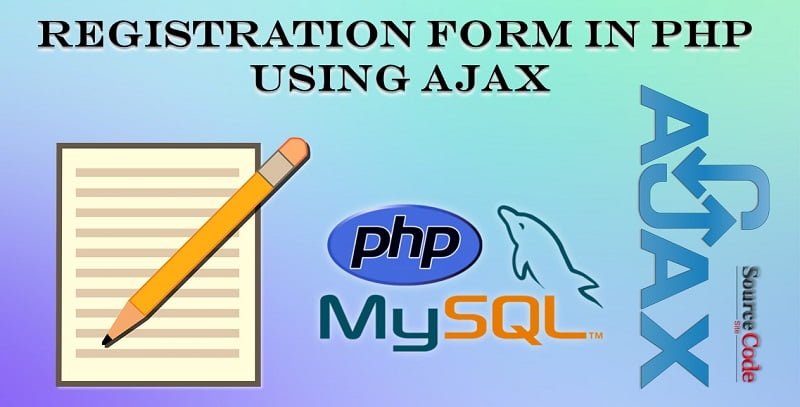
In this article we are going to teach you how to create registration form in PHP using ajax and delete data from font-end. This tutorial will help you to learn how to register any user for your website. In user registration, we collect their information and store it in a MySQL database.
How to Create Registration Form in PHP using Ajax
1-Creating Database
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “register”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.7.0 -- https://www.phpmyadmin.net/ -- -- Host: 127.0.0.1 -- Generation Time: Oct 06, 2017 at 09:15 AM -- Server version: 10.1.25-MariaDB -- PHP Version: 5.6.31 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Database: `register` -- -- -------------------------------------------------------- -- -- Table structure for table `user` -- CREATE TABLE `user` ( `userid` int(11) NOT NULL, `firstname` varchar(30) NOT NULL, `lastname` varchar(30) NOT NULL, `username` varchar(30) NOT NULL, `password` varchar(30) NOT NULL, `address` varchar(100) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `user` -- INSERT INTO `user` (`userid`, `firstname`, `lastname`, `username`, `password`, `address`) VALUES (46, 'Deepak', 'Raj', 'deepakraj', '123456789', 'India'), (47, 'Akash', 'kumar', 'example@mail.com', '123456', 'India'); -- -- Indexes for dumped tables -- -- -- Indexes for table `user` -- ALTER TABLE `user` ADD PRIMARY KEY (`userid`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `user` -- ALTER TABLE `user` MODIFY `userid` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=48;COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder.
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “register”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find Database folder in Downloaded source code where find register.sql file then Select it.
- Then Click on Go.
2- Creating Database Connection
After import Database File then next step is creating database connection using php copy the below code and save it is as “conn.php”.
<?php $conn = mysqli_connect("localhost","root","","register"); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } ?>
3 – Creating Form For Register Data
in this step we are going to create a form for register data .
<!DOCTYPE html> <html> <head> <title>Registration Form in PHP using Ajax</title> <script src="js/jquery.min.js"></script> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" /> <script src="js/bootstrap.min.js"></script> <link rel="stylesheet" href="css/style.css" /> </head> <body> <div class="container"> <div class="h20"></div> <div class="well main"> <div class="row"> <div class="col-lg-12"> <span class="title"><center>Registration Form in PHP using Ajax<hr style="border-top: 3px double #8c8b8b;"></center></span> </div> </div> <div class="h20"></div> <form id="form"> <div class="row"> <div class="col-lg-2"> <span class="desc">First Name:</span> </div> <div class="col-lg-10"> <input type="text" name="firstname" class="form-control"> </div> </div> <div class="h10"></div> <div class="row"> <div class="col-lg-2"> <span class="desc">Last Name:</span> </div> <div class="col-lg-10"> <input type="text" name="lastname" class="form-control"> </div> </div> <div class="h10"></div> <div class="row"> <div class="col-lg-2"> <span class="desc">Username:</span> </div> <div class="col-lg-10"> <input type="text" name="username" class="form-control"> </div> </div> <div class="h10"></div> <div class="row"> <div class="col-lg-2"> <span class="desc">Password:</span> </div> <div class="col-lg-10"> <input type="password" name="password" class="form-control"> </div> </div> <div class="h10"></div> <div class="row"> <div class="col-lg-2"> <span class="desc">Address:</span> </div> <div class="col-lg-10"> <input type="text" name="address" class="form-control"> </div> </div> </form> <div class="h10"></div> <div class="row"> <div class="col-lg-12"> <button type="button" class="btn btn-success pull-right" id="submit">Register Now</button> </div> </div> </div> <div class="h20"></div> <div class="row"> <div id="table"> </div> </div> </div> <script src="js/custom.js"></script> </body> </html>
4 – Give Some Style For Looking Good using CSS
.title{ font-size:30px; color:#D104BB; } .main{ width:60%; padding:auto; margin:auto; } .h20{ height:20px; } .h10{ height:10px; } .desc{ position:relative; top:6px; } input[type="text"]{ border:1px solid #000; } input[type="password"]{ border:1px solid #000; } button[type="button"]{ background-color: #665564 ; border:1px solid #000; } input:focus { background-color: #665564 ; color:#fff; border:1px dotted #000; }
5 – Inserting Data into Database using PHP & Ajax
Next step is inserting data into database using PHP MySQL and Ajax save it as add.php.
<?php include('conn.php'); if(isset($_POST['firstname'])){ $firstname=$_POST['firstname']; $lastname=$_POST['lastname']; $username=$_POST['username']; $password=$_POST['password']; $address=$_POST['address']; mysqli_query($conn,"insert into user (firstname, lastname, username, password, address) values ('$firstname', '$lastname', '$username', '$password', '$address')"); } ?>
6 – Display, Delete Data using PHP and Ajax
in this step we are fetching data from MySQL Database using PHP and ajax.
<?php include('conn.php'); if(isset($_POST['fetch'])){ ?> <table class="table table-bordered table-striped"> <thead> <th>Firstname</th> <th>Lastname</th> <th>Username</th> <th>Password</th> <th>Address</th> <th>Delete</th> </thead> <tbody> <?php $query=mysqli_query($conn,"select * from user order by userid desc"); while($row=mysqli_fetch_array($query)){ ?> <tr> <td><?php echo $row['firstname']; ?></td> <td><?php echo $row['lastname']; ?></td> <td><?php echo $row['username']; ?></td> <td><?php echo $row['password']; ?></td> <td><?php echo $row['address']; ?></td> <td><a href="delete.php?userid=<?php echo md5($row['userid']);?>"><button type="button" class="btn btn-danger">Delete</button> </a> </td> </tr> <?php } ?> </tbody> </table> <?php } ?> <script type="text/javascript"> $(document).ready(function() { $('.btn-danger').click(function() { var id = $(this).attr("id"); if (confirm("Are you sure you want to delete this Member?")) { $.ajax({ type: "POST", url: "delete_member.php", data: ({ id: id }), cache: false, success: function(html) { $(".delete_mem" + id).fadeOut('slow'); } }); } else { return false; } }); }); </script>
7 – Create Delete Function using PHP
in this step we are creating a file named delete.php.
<?php include 'conn.php'; $id = $_GET['userid']; $sql = "Delete from user where md5(userid) = '$id'"; if($conn->query($sql) === true){ echo "Sucessfully deleted data"; header('location:index.php'); }else{ echo "Oppps something error "; } $conn->close(); ?>
8 – Custom Js For Add Registration and Fetch Data
$(document).ready(function(){ showTable(); $('#submit').click(function(){ var form=$('#form').serialize(); $.ajax({ url:"add.php", method:"POST", data:form, success:function(){ showTable(); $('#form')[0].reset(); } }); }); }); function showTable(){ $.ajax({ url:"fetch.php", method:"POST", data:{ fetch: 1, }, success:function(data){ $('#table').html(data); } }); }
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.