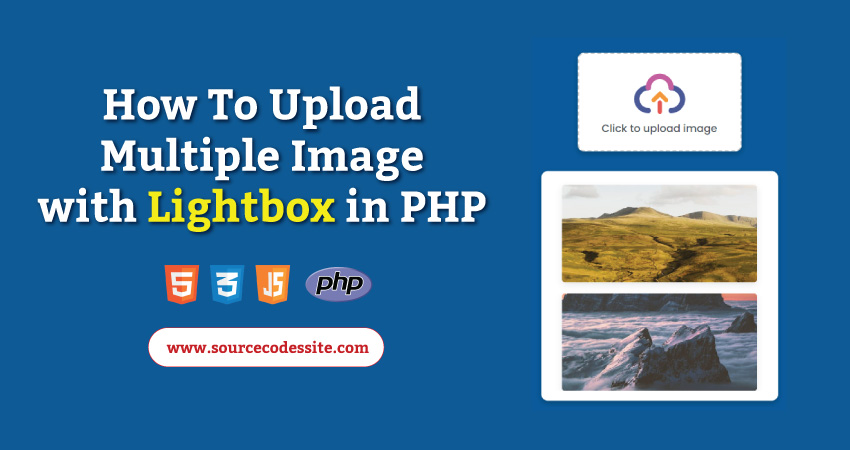
How To Upload Multiple Image with Lightbox in PHP
To enable Upload Multiple Image with a lightbox effect in PHP, create an HTML form allowing users to select and submit multiple images. Implement a PHP backend to handle file uploads, moving the files to a designated server folder.
Display the uploaded images on the webpage using PHP, and wrap each image link in an anchor tag, setting the href attribute to the image file path. Integrate a lightweight JavaScript lightbox library, such as baguetteBox.js, into the HTML, and initialize it with the appropriate class selector for the image container.
This combined PHP and JavaScript solution provides users with a seamless experience, allowing them to upload multiple images and view them in an aesthetically pleasing lightbox gallery. Enhance the interface with features like image previews before uploading for a more interactive and user-friendly image upload process.
Realted Source Code
- How To Upload Multiple Files using AngularJS with PHP/MySQLi
- How to upload image without database using PHP
- How to upload Multiple image in PHP MySQL Using Ajax
Demo
Follow This Step to Create Upload Multiple Image with Lightbox in PHP
1 – Creating Index Page
Create a sleek HTML and CSS index page for multiple image uploads with PHP backend. The page includes an intuitive form allowing users to select and submit multiple images. PHP handles the file upload functionality, storing images in a designated server folder. The uploaded images are dynamically displayed on the page, and each image link is wrapped in an anchor tag, facilitating integration with a JavaScript lightbox library. The user experience is enhanced with features like image previews before uploading and a stylish interface, providing a seamless and visually appealing image upload process. This concise solution ensures an efficient and engaging experience for users interacting with the image gallery. create index page into your project folder then copy below code and paste into index.php file.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Upload Multiple Image in PHP</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/baguettebox.js/1.11.0/baguetteBox.min.css"> <link rel="stylesheet" href="css/style.css"> </head> <body> <div class="main"> <div class="container"> <div class="header-container"> <h1>Upload Multiple Image in PHP</h1> <form action="./includes/upload.php" method="POST" enctype="multipart/form-data"> <label class="custom-file-upload" for="file"> <div class="icon"> <svg viewBox="0 0 1024 1024" class="icon" version="1.1" xmlns="http://www.w3.org/2000/svg" fill="#000000"><g id="SVGRepo_bgCarrier" stroke-width="0"></g><g id="SVGRepo_tracerCarrier" stroke-linecap="round" stroke-linejoin="round"></g><g id="SVGRepo_iconCarrier"><path d="M736.68 435.86a173.773 173.773 0 0 1 172.042 172.038c0.578 44.907-18.093 87.822-48.461 119.698-32.761 34.387-76.991 51.744-123.581 52.343-68.202 0.876-68.284 106.718 0 105.841 152.654-1.964 275.918-125.229 277.883-277.883 1.964-152.664-128.188-275.956-277.883-277.879-68.284-0.878-68.202 104.965 0 105.842zM285.262 779.307A173.773 173.773 0 0 1 113.22 607.266c-0.577-44.909 18.09-87.823 48.461-119.705 32.759-34.386 76.988-51.737 123.58-52.337 68.2-0.877 68.284-106.721 0-105.842C132.605 331.344 9.341 454.607 7.379 607.266 5.417 759.929 135.565 883.225 285.262 885.148c68.284 0.876 68.2-104.965 0-105.841z" fill="#4A5699"></path><path d="M339.68 384.204a173.762 173.762 0 0 1 172.037-172.038c44.908-0.577 87.822 18.092 119.698 48.462 34.388 32.759 51.743 76.985 52.343 123.576 0.877 68.199 106.72 68.284 105.843 0-1.964-152.653-125.231-275.917-277.884-277.879-152.664-1.962-275.954 128.182-277.878 277.879-0.88 68.284 104.964 68.199 105.841 0z" fill="#C45FA0"></path><path d="M545.039 473.078c16.542 16.542 16.542 43.356 0 59.896l-122.89 122.895c-16.542 16.538-43.357 16.538-59.896 0-16.542-16.546-16.542-43.362 0-59.899l122.892-122.892c16.537-16.542 43.355-16.542 59.894 0z" fill="#F39A2B"></path><path d="M485.17 473.078c16.537-16.539 43.354-16.539 59.892 0l122.896 122.896c16.538 16.533 16.538 43.354 0 59.896-16.541 16.538-43.361 16.538-59.898 0L485.17 532.979c-16.547-16.543-16.547-43.359 0-59.901z" fill="#F39A2B"></path><path d="M514.045 634.097c23.972 0 43.402 19.433 43.402 43.399v178.086c0 23.968-19.432 43.398-43.402 43.398-23.964 0-43.396-19.432-43.396-43.398V677.496c0.001-23.968 19.433-43.399 43.396-43.399z" fill="#E5594F"></path></g></svg> </div> <div class="text"> <span>Click to upload image</span> </div> <input type="file" name="file[]" id="file" multiple> </label> <div class="preview-container" style="display: none;"> <!-- Generated by Script --> </div> <button type="submit" class="upload-button" style="display: none;">Upload Image</button> </form> </div> <div class="uploaded-image-container"> <h2>Uploaded Images:</h2> <div class="images"> <?php $uploadDir = 'uploads/'; $imageFiles = glob($uploadDir . '*.{jpg,jpeg,png,gif}', GLOB_BRACE); foreach ($imageFiles as $imageFile) { $imageName = basename($imageFile); echo '<a href="'. $uploadDir . $imageName . '" data-lightbox="uploaded-images"><img src="' . $uploadDir . $imageName . '" alt="' . $imageName . '"></a>'; } ?> </div> </div> </div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/baguettebox.js/1.11.0/baguetteBox.min.js"></script> <script src="js/script.js"></script> </body> </html>
2 – Image Validation using PHP
Create Upload.php File Under Include Folder.
The script checks if the form has been submitted using the POST method. If submitted, it defines the target upload directory and creates it if it doesn’t exist. It then processes each uploaded file:
- Image Validation: It checks if the uploaded file is a valid image by using getimagesize.
- Duplicate Check: Verifies if the file with the same name already exists in the upload directory.
- File Size Check: Ensures the file size is within the allowed limit (5MB).
- File Format Check: Only allows specific image formats (jpg, jpeg, png, gif).
- Move Uploaded File: If all checks pass, the script moves the file from the temporary location to the upload directory.
Upon successful upload, the user receives a success alert and is redirected to the upload page. If any validation fails, appropriate error alerts are displayed, and the user is redirected to the upload page. copy below code and paste into upload.php file.
<?php // Check if the form is submitted if ($_SERVER['REQUEST_METHOD'] === 'POST') { // Define the upload directory $uploadDir = '../uploads/'; // Create the upload directory if it doesn't exist if (!file_exists($uploadDir)) { mkdir($uploadDir, 0777, true); } // Process each uploaded file foreach ($_FILES['file']['name'] as $key => $filename) { $targetFile = $uploadDir . basename($filename); $imageFileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION)); // Check if the file is an actual image $check = getimagesize($_FILES['file']['tmp_name'][$key]); if ($check === false) { echo " <script> alert('File is not an image!'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; exit; } // Check if the file already exists if (file_exists($targetFile)) { echo " <script> alert('File already exist!'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; exit; } // Check file size if ($_FILES['file']['size'][$key] > 5000000) { echo " <script> alert('The file is too large. The maximum size is 5mb'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; exit; } // Allow only certain file formats $allowedFormats = ['jpg', 'jpeg', 'png', 'gif']; if (!in_array($imageFileType, $allowedFormats)) { echo " <script> alert('Only img, jpeg, png and gif is allowed.'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; exit; } // Move the file to the upload directory if (move_uploaded_file($_FILES['file']['tmp_name'][$key], $targetFile)) { echo " <script> alert('Images uploaded successfully.'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; } else { echo " <script> alert('Error Uploading Images.'); window.location.href = 'http://localhost/multiple-image-upload/'; </script> "; } } } else { // If the form is not submitted, return an error echo 'Invalid request.'; }
3 – Styling All Elements Using CSS
Apply CSS styles consistently across all pages to create a visually appealing and user-friendly interface. Use responsive design principles to ensure a good user experience on various devices. you can create a css folder under project then create s style.css after that copy paste the below code.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@500&display=swap'); * { margin: 0; padding: 0; font-family: 'Poppins', sans-serif; } body { background-image: linear-gradient(to top, #cfd9df 0%, #e2ebf0 100%); height: 100vh; } .main { display: flex; justify-content: center; align-items: center; } .header-container > form { display: flex; flex-direction: column; align-items: center; } .header-container { text-align: center; } .header-container > h1 { font-size: 50px; margin: 30px; text-shadow: 2px 2px rgb(200, 200, 200); } .custom-file-upload { height: 100px; width: 200px; display: flex; flex-direction: column; align-items: center; justify-content: center; cursor: pointer; border: 2px dashed #cacaca; background-color: rgba(255, 255, 255, 1); padding: 1.5rem; border-radius: 10px; box-shadow: rgba(0, 0, 0, 0.24) 0px 3px 8px; } .custom-file-upload .icon { display: flex; align-items: center; justify-content: center; } .custom-file-upload .icon svg { height: 80px; } .custom-file-upload .text { display: flex; align-items: center; justify-content: center; } .custom-file-upload .text span { font-weight: 400; color: rgba(75, 85, 99, 1); } .custom-file-upload input { display: none; } .preview-container img { max-width: 100%; height: auto; margin: 10px; border-radius: 8px; box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1); } .preview-container { display: flex; justify-content: center; flex-wrap: wrap; max-width: 1000px; box-shadow: rgba(0, 0, 0, 0.24) 0px 3px 8px; padding: 20px; border-radius: 10px; margin: 50px; background-color: rgba(255, 255, 255, 1); } .preview-container > img { height: 100px; } .upload-button { padding: 10px; width: 200px; border-radius: 10px; border: none; font-size: 15px; background-color: rgba(255, 255, 255, 1); box-shadow: rgba(0, 0, 0, 0.16) 0px 1px 4px; cursor: pointer; } .uploaded-image-container { display: flex; flex-direction: column; width: 1300px; box-shadow: rgba(0, 0, 0, 0.24) 0px 3px 8px; padding: 20px; border-radius: 10px; background-color: rgba(255, 255, 255, 1); margin-top: 30px; height: 500px; } .uploaded-image-container > h2 { margin: 10px; text-shadow: 2px 2px rgb(200, 200, 200); } .images { display: flex; flex-wrap: wrap; justify-content: center; overflow-y: auto; } .images a > img { height: 150px; width: 300px; margin: 5px; object-fit: cover; border-radius: 5px; box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1); transition: transform 0.3s ease-in-out; cursor: pointer; } .images a > img:hover { transform: scale(1.05); }
4 – Validate Elements Using JavaScript
Implement JavaScript to validate certain form inputs on the client side, providing immediate feedback to users and reducing server load. This can include password strength validation or email format checks. Create a “js” folder in the project and add a “script.js” file. Copy and paste the provided code.
baguetteBox.run('.images', { animation: 'fadeIn' }); document.getElementById('file').addEventListener('change', handleFileSelect); function handleFileSelect(event) { const previewContainer = document.querySelector('.preview-container'); const uploadedImageContainer = document.querySelector('.uploaded-image-container'); const uploadButton = document.querySelector('.upload-button'); previewContainer.style.display = ''; uploadButton.style.display = ''; uploadedImageContainer.style.display = 'none'; const files = event.target.files; for (const file of files) { const reader = new FileReader(); reader.onload = function (e) { const image = document.createElement('img'); image.src = e.target.result; previewContainer.appendChild(image); }; reader.readAsDataURL(file); } }
Now Run your project on browser . if any problem with this Download the Complete source code in zip Formate by clicking the below button Download Source Code otherwise you can send Comment.
5 – Download Source Code
6 – conclusion
In conclusion, implementing a multiple-image upload with Lightbox in PHP enhances the user experience by allowing seamless image browsing and viewing. The combination of PHP for server-side processing and Lightbox for an interactive image gallery creates a dynamic and visually appealing solution. Users can effortlessly upload, preview, and view multiple images within a sleek and responsive interface. This approach not only improves the functionality of image uploads but also adds a layer of sophistication to the overall web experience. With the added benefit of Lightbox, users can conveniently navigate through uploaded images in a visually engaging manner. This integration is a practical and aesthetically pleasing solution for web applications that require efficient multiple-image handling.