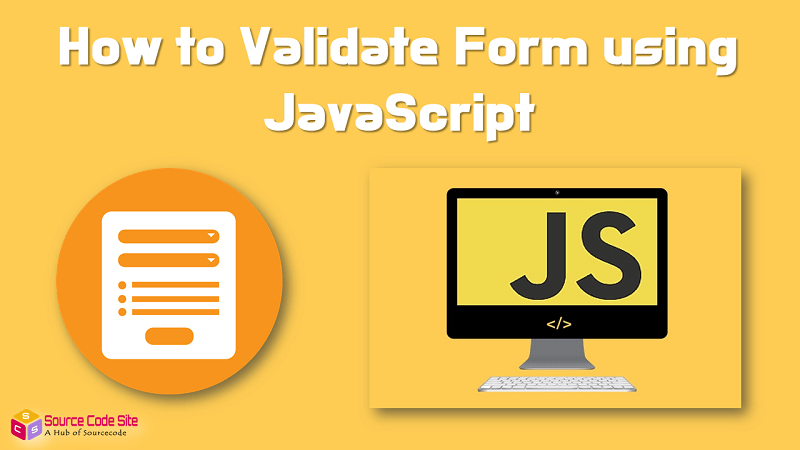
How to validate Form using JavaScript
Javascript provide a way to validate form data on the clients’s computer before sending it to the web server. Form validation normally used to occur at the server after the client had entered all necessary data and then pressed the submit button. When you create form’s providing form validation is useful to ensure that your viewer enter valid and complete data. For example, you may want to ensure that someone inserts a valid e-mail address into a text box, or perhaps you want to ensure that someone fill in certain fields. If the data entered by a client was incorrect or was simply missing, the server would have to send all the data back to the client and request that the form be resubmitted with correct information.
How to validate Form using JavaScript
The javascript provides you the facility the validate the form on the client side so processing will fast than server side validation. So most of the web developers prefer javascript form validation.
Creating Form using HTML
To get started you need a form and in this step we are going to create a form using HTML. For purpose of this article , paste the following source code into a newpage in text editor.
<form id="theForm" method="get" action="submitData" > <table id="font"> <tr> <td width="168">Name<span class="red">*</span></td> <td width="288"><input type="text" id="name" name="name" class="input1"/></td> <td width="183" class="red" id="nameError"> </td> </tr> <tr> <td>Address</td> <td><input type="email" id="address" class="input1" /></td> <td id="addressError" class="red"> </td> </tr> <tr> <td>Pin Code<span class="red">*</span></td> <td><input type="text" id="zipcode" name="zipcode" class="input1"/></td> <td id="zipcodeError" class="red"> </td> </tr> <tr> <td>Country<span class="red">*</span></td> <td><select id="country" name="country" class="input1"> <option value="" selected>Please select...</option> <option value="India">India</option> <option value="Pakistan">Pakistan</option> <option value="Afganistan">Afganistan</option> <option value="Australia">Australia</option> </select><br /></td> <td id="countryError" class="red"> </td> </tr> <tr> <td>Gender<span class="red">*</span></td> <td><p> <input type="radio" name="gender" value="m" /> Male <input type="radio" name="gender" value="f" /> Female</p> </td> <td id="genderError" class="red"> </td> </tr> <tr> <td>Preferences<span class="red">*</span></td> <td><p> <input type="checkbox" name="color" value="r" /> Red <input type="checkbox" name="color" value="g" /> Green <input type="checkbox" name="color" value="b" /> Blue</p> </td> <td id="colorError" class="red"> </td> </tr> <tr> <td>Phone<span class="red">*</span></td> <td><input type="text" id="phone" name="phone" class="input1"/></td> <td id="phoneError" class="red"> </td></tr> <tr> <td>Email<span class="red">*</span></td> <td><input type="text" id="email" name="email" class="input1"/></td> <td id="emailError" class="red"> </td> </tr> <tr> <td>password <span class="red">*</span></td> <td><input type="password" id="password" name="password" class="input1"/></td> <td id="passwordError" class="red"> </td> </tr> <tr> <td>Verify password<span class="red">*</span></td> <td><input type="password" id="pwVerified" name="pwVerified" class="input1"/></td> <td id="pwVerifiedError" class="red"> </td> </tr> <tr> <td><input name="submit" type="submit" class="input1" id="submit" value="Submit"/></td> <td><p> <input name="reset" type="reset" class="input1" id="reset" value="Reset"/> </p></td> <td> </td></tr> </table> </form>
Styling The Form using CSS
in this step we going to give style for the Form using CSS.
html, body, div, span, applet, object, iframe, h1, h2, h3, h4, h5, h6, p, blockquote, pre, a, abbr, acronym, address, big, cite, code, del, dfn, em, img, ins, kbd, q, s, samp, small, strike, strong, sub, sup, tt, var, b, u, i, center, dl, dt, dd, ol, ul, li, fieldset, form, label, legend, table, caption, tbody, tfoot, thead, tr, th, td, article, aside, canvas, details, embed, figure, figcaption, footer, header, hgroup, menu, nav, output, ruby, section, summary, time, mark, audio, video { margin: 0; padding: 0; } /* HTML5 display-role reset for older browsers */ article, aside, details, figcaption, figure, footer, header, hgroup, menu, nav, section { display: block; } body { line-height: 1; background-color:skyblue; } .red { color: red; } .input1{ width:200px; height:30px; padding:0px; border:1px solid red; border-radius:5px; } input.error{ width:200px; height:30px; padding:0px; border:2px solid red; border-radius:5px; } table { border:0; } td { margin: 0; padding: 3px 10px 3px 3px; } #font{ font-size:18; color:black }
Validate Form using Javascript
You need a custom form that you can later connect to the form. A function is a piece of code that consists of one or more line of script. A function can take arguments and can return values. The validation script in this post returns a boolean value that specifies whether tha data contained in the form is valid.
window.onload = init; function init() { document.getElementById("theForm").onsubmit = validateForm; document.getElementById("reset").onclick = clearDisplay; document.getElementById("name").focus(); } function validateForm() { return (isNotEmpty("name", "Please enter your name!") && isNumeric("zipcode", "Please enter a 5-digit zip code!") && isLengthMinMax("zipcode", "Please enter a 5-digit zip code!", 5, 5) && isSelected("country", "Please make a selection!") && isChecked("gender", "Please check a gender!") && isChecked("color", "Please check a color!") && isNumeric("phone", "Please enter a valid phone number!") && isValidEmail("email", "Enter a valid email!") && isLengthMinMax("password", "Enter a valid password!", 6, 8) && verifyPassword("password", "pwVerified", "Different from the password!")); } function isNotEmpty(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value.trim(); var isValid = (inputValue.length !== 0); // boolean showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function showMessage(isValid, inputElement, errorMsg, errorElement) { if (!isValid) { if (errorElement !== null) { errorElement.innerHTML = errorMsg; } else { alert(errorMsg); } if (inputElement !== null) { inputElement.className = "error"; inputElement.focus(); } } else { if (errorElement !== null) { errorElement.innerHTML = ""; } if (inputElement !== null) { inputElement.className = ""; } } } function isNumeric(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value.trim(); var isValid = (inputValue.search(/^[0-9]+$/) !== -1); showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isAlphabetic(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value.trim(); var isValid = inputValue.match(/^[a-zA-Z]+$/); showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isAlphanumeric(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value.trim(); var isValid = inputValue.match(/^[0-9a-zA-Z]+$/); showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isLengthMinMax(inputId, errorMsg, minLength, maxLength) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value.trim(); var isValid = (inputValue.length >= minLength) && (inputValue.length <= maxLength); showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isValidEmail(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value; var atPos = inputValue.indexOf("@"); var dotPos = inputValue.lastIndexOf("."); var isValid = (atPos > 0) && (dotPos > atPos + 1) && (inputValue.length > dotPos + 2); showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isSelected(inputId, errorMsg) { var inputElement = document.getElementById(inputId); var errorElement = document.getElementById(inputId + "Error"); var inputValue = inputElement.value; var isValid = inputValue !== ""; showMessage(isValid, inputElement, errorMsg, errorElement); return isValid; } function isChecked(inputName, errorMsg) { var inputElements = document.getElementsByName(inputName); var errorElement = document.getElementById(inputName + "Error"); var isChecked = false; for (var i = 0; i < inputElements.length; i++) { if (inputElements[i].checked) { isChecked = true; break; } } showMessage(isChecked, null, errorMsg, errorElement); return isChecked; } function verifyPassword(pwId, verifiedPwId, errorMsg) { var pwElement = document.getElementById(pwId); var verifiedPwElement = document.getElementById(verifiedPwId); var errorElement = document.getElementById(verifiedPwId + "Error"); var isTheSame = (pwElement.value === verifiedPwElement.value); showMessage(isTheSame, verifiedPwElement, errorMsg, errorElement); return isTheSame; } function clearDisplay() { var elms = document.getElementsByTagName("*"); for (var i = 0; i < elms.length; i++) { if ((elms[i].id).match(/Error$/)) { elms[i].innerHTML = ""; } if (elms[i].className === "error") { elms[i].className = ""; } } document.getElementById("name").focus(); }
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.