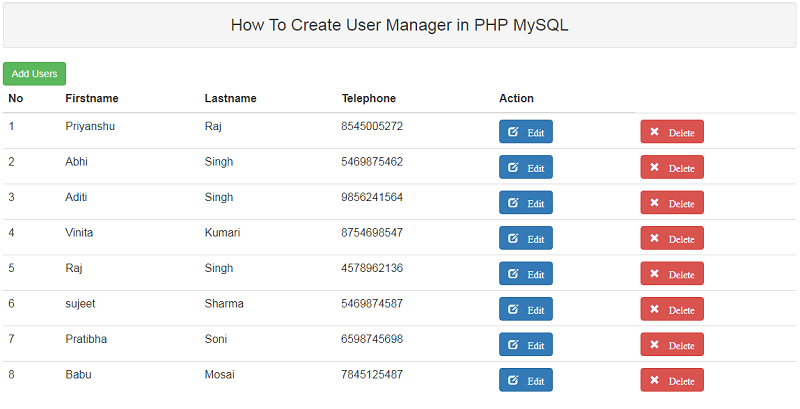
How To Create User Manager in PHP MySQL in this user manager we used CRUD operation. Creating CRUD is a very common task in web development CRUD stand For Create, Read, Update and Delete. if you are a senior web developer you must have created many of CRUD system already. They maybe exist in a content management system, and inventory management system OR accounting application. if you just started web development, you are certainly going to experience lots CRUD grid’s creation work in your later career. The main purpose of a CRUD system is that enables users create, read, update, and delete data. Normally data stored in MySQL Database. PHP is the server side scripting language that manipulates MySQL Database tables to give Front end users power to perform CRUD action.
How To Create User Manager in PHP MySQL
1-Creating Database
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “users”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.7.0 -- https://www.phpmyadmin.net/ -- -- Host: 127.0.0.1 -- Generation Time: Jan 22, 2018 at 11:23 AM -- Server version: 10.1.25-MariaDB -- PHP Version: 5.6.31 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Database: `users` -- -- -------------------------------------------------------- -- -- Table structure for table `tasks` -- CREATE TABLE `tasks` ( `id` int(12) NOT NULL, `firstname` text NOT NULL, `lastname` text NOT NULL, `phone` varchar(15) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `tasks` -- INSERT INTO `tasks` (`id`, `firstname`, `lastname`, `phone`) VALUES (3, 'Priyanshu', 'Raj', '8545005272'), (4, 'Abhi', 'Singh', '5469875462'), (5, 'Aditi', 'Singh', '9856241564'), (6, 'Vinita', 'Kumari', '8754698547'), (7, 'Raj', 'Singh', '4578962136'), (9, 'sujeet', 'Sharma', '5469874587'), (11, 'Pratibha', 'Soni', '6598745698'), (12, 'Babu', 'Mosai', '7845125487'); -- -- Indexes for dumped tables -- -- -- Indexes for table `tasks` -- ALTER TABLE `tasks` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `tasks` -- ALTER TABLE `tasks` MODIFY `id` int(12) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=13;COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder where found db folder to access db file.
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “users”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find Db file in Downloaded source code Select it.
- Then Click on Go.
2- Creating Database Connection
After import Database File then next step is creating database connection using php copy the below code and save it is as “config.php”.
<?php define('DB_HOST', 'localhost'); define('DB_USER', 'root'); define('DB_PASS', ''); define('DB_NAME', 'users');
3 – Creating Index Page To Manage All Data
This step we are creating a page to manage all users easily named index.php. Source code are given below.
<?php include_once('./config/Config.php'); include_once('./lib/Database.php'); include_once('./inc/header.php'); ?> <?php $db = new Database(); $query = "SELECT * FROM tasks"; $read = $db->Select($query); ?> <div class="container"> <h3 class="well text-center">How To Create User Manager in PHP MySQL</h3> <table class="table"> <thead class="thead"> <tr> <th>No</th> <th>Firstname</th> <th>Lastname</th> <th>Telephone</th> <th>Action</th> </tr> </thead> <tbody> <?php $i = 1; ?> <?php if($read) { ?> <?php while($row = $read->fetch_assoc()) { ?> <tr> <td><?php echo $i++; ?></td> <td><?php echo $row['firstname']; ?></td> <td><?php echo $row['lastname'];?></td> <td><?php echo $row['phone']; ?></td> <td><a href="update.php?id=<?php echo $row['id']; ?>" class="glyphicon glyphicon-edit btn btn-primary"> Edit</a></td> <td><a href="delete.php?id=<?php echo $row['id']; ?>" class="glyphicon glyphicon-remove btn btn-danger"> Delete</a></td> </tr> <?php } ?> <?php }else{ echo "DATA NOT FOUND"; } ?> </tbody> <span><a href="create.php" class="btn btn-success">Add Users</a></span> </table> </div>
4 – Create Form and Send Data into MySQL Database using PHP
This step we are creating a page named create.php here we are create a form in simple html and insert data into mysql database using php.
<?php include_once('./config/Config.php'); include_once('./lib/Database.php'); include_once('./inc/header.php'); ?> <div class="container"> <h3 class="well text-center">How To Create User Manager in PHP MySQL</h3> <?php $db = new Database(); // check the form if(isset($_POST['submit'])) { $firstname= mysqli_real_escape_string($db->link, $_POST['firstname']); $lastname = mysqli_real_escape_string($db->link, $_POST['lastname']); $phone = mysqli_real_escape_string($db->link, $_POST['phone']); if($firstname == '' || $lastname == '' || $phone == ''){ $errors = "<div class='alert alert-danger'> the fields can not be empty"."</div>"; echo $errors; }else{ // insert into the database table $sql = "INSERT INTO tasks(firstname, lastname, phone) VALUES('$firstname', '$lastname', '$phone')"; $createAll = $db->Insert($sql); } } ?> <hr> <span><a href="index.php" class="btn btn-success">Go Back</a></span> <div style="max-width:500px; margin: 0 auto;"> <form action="create.php" method="post"> <div class="form-group"> <label for="Firstname">Firstname</label> <input type="text" name="firstname" value="" class="form-control"> </div> <div class="form-group"> <label for="Firstname">Lastname</label> <input type="text" name="lastname" class="form-control"> </div> <div class="form-group"> <label for="Firstname">Telephone</label> <input type="number" name="phone" placeholder="numbers only" class="form-control"> </div> <div class="form-group"> <input type="submit" name="submit" class="btn btn-primary" value="Add"> <input type="reset" name="reset" class="btn btn-success" value="Cancel"> </div> </form> </div> </div>
5 – Creating Update Form and Validate using PHP
This step we are update inserted data into mysql using php.
<?php include_once('./config/Config.php'); include_once('./lib/Database.php'); include_once('./inc/header.php'); ?> <div class="container"> <h3 class="well text-center">How To Create User Manager in PHP MySQL</h3> <?php $db = new Database(); $id = $_GET['id']; $getid = "SELECT * FROM tasks WHERE id=$id"; $query_id = $db->Select($getid)->fetch_assoc(); // check the form if(isset($_POST['submit'])) { $firstname= mysqli_real_escape_string($db->link, $_POST['firstname']); $lastname = mysqli_real_escape_string($db->link, $_POST['lastname']); $phone = mysqli_real_escape_string($db->link, $_POST['phone']); if($firstname == '' || $lastname == '' || $phone == ''){ $errors = "<div class='alert alert-danger'> the fields can not be empty"."</div>"; echo $errors; }else{ // update the database table $sql = "UPDATE tasks SET firstname ='$firstname', lastname='$lastname', phone='$phone' WHERE id=$id"; $update_id = $db->Update($sql); } } ?> <hr> <span><a href="index.php" class="btn btn-success">Go Back</a></span> <div style="max-width:500px; margin: 0 auto;"> <form action="" method="post"> <div class="form-group"> <label for="Firstname">Firstname</label> <input type="text" name="firstname" value="<?php echo $query_id['firstname']; ?>" class="form-control"> </div> <div class="form-group"> <label for="Firstname">Lastname</label> <input type="text" name="lastname" value="<?php echo $query_id['lastname']; ?>" class="form-control"> </div> <div class="form-group"> <label for="Firstname">Telephone</label> <input type="number" name="phone" value="<?php echo $query_id['phone']; ?>" placeholder="numbers only" class="form-control"> </div> <div class="form-group"> <input type="submit" name="submit" class="btn btn-primary" value="update"> <!-- <input type="reset" name="reset" class="btn btn-success" value="Cancel"> --> </div> </form> </div> </div>
6 – Create Delete Function using PHP
This Function use for delete data from mysql database using php.
<?php include_once('./config/Config.php'); include_once('./lib/Database.php'); include_once('./inc/header.php'); ?> <?php $db = new Database(); $id = $_GET['id']; $delete = "DELETE FROM tasks WHERE id=$id"; $deleteID = $db->Delete($delete); ?> <h4 class="text-muted center"><a href="index.php"> GO back...</h4></p>
7 – Creating Error Function using PHP
This step we showing alert after fetching any types of error.
<?php class Database { public $host = DB_HOST; public $user = DB_USER; public $pass = DB_PASS; public $dbname = DB_NAME; public $link; public $error; public function __construct(){ $this->connectDB(); } private function connectDB(){ $this->link = new mysqli($this->host, $this->user, $this->pass, $this->dbname); if(!$this->link){ $this->error= "connection failed". $this->link->connect_error; } } // read or select data public function Select($query){ $result = $this->link->query($query) or die($this->link->error. __LINE__); if($result->num_rows > 0){ return $result; }else{ return false; } } public function Insert($query){ $insert_row = $this->link->query($query) or die($this->link->error. __LINE__); if($insert_row){ $success = "<h4 class = 'alert alert-success'>Inserted successfully!"."</h4>"; echo $success; }else{ $this->link->error; } } public function Update($query){ $update_id = $this->link->query($query) or die($this->link->error.__LINE__); if($update_id){ $success = "<h4 class = 'alert alert-success'>Data Inserted successfully!"."</h4>"; echo $success; }else{ $this->link->error; } } public function Delete($query){ $delete_id = $this->link->query($query) or die($this->link->error.__LINE__); if($delete_id){ $success = "<h4 class = 'alert alert-success'>Deleted successfully!"."</h4>"; echo $success; } } }
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.
Very nicee
whoah this blog is magnificent i really like reading your posts.
Keep up the great work! You already know, many persons are looking round for this info, you can aid them greatly.