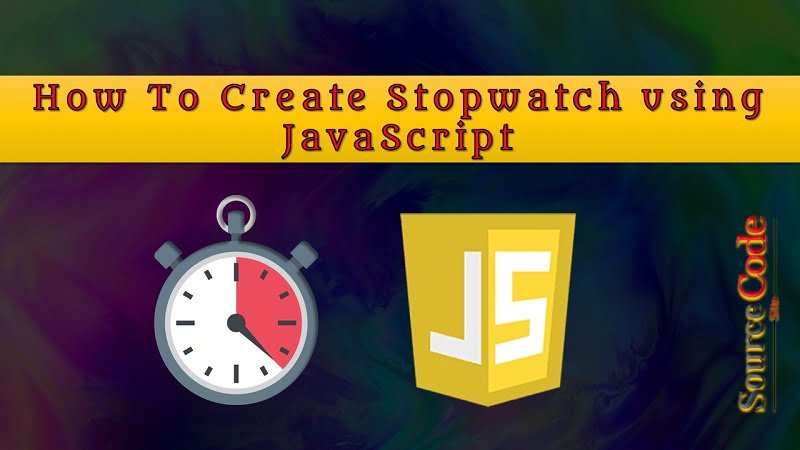
How To Create Stopwatch using JavaScript
Hello, friends In this post I am going to teach you how to make a stopwatch in JavaScript. In which you will learn how to make a stopwatch with the help of three buttons, which will have to start, stop and a reset button. JavaScript stopwatch or rather stop down timer can be implemented using the JavaScript timing methods which are executed in time intervals.
JavaScript timing is made up of the following methods –
setTimeout( “javascript expression”, milliseconds );
clearTimeout( ID of setTimeout( ) );
When using the JavaScript timing methods, you need to understand how JavaScript executes statements in time intervals.
In creating a stopwatch, we have used bootstrap and google font CDN to create layouts.
In this step, we have created a stopwatch and created three buttons so that the stopwatch will be controlled.
<!DOCTYPE html> <html lang="en"> <head> <title>How To Create Stopwatch using JavaScript</title> <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/> <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/> <link href="https://fonts.googleapis.com/css?family=Lobster" rel="stylesheet"> <style type="text/css"> body{margin-top:150px;} .well{ -webkit-box-shadow: 9px 7px 52px -6px rgba(133,133,133,1); -moz-box-shadow: 9px 7px 52px -6px rgba(133,133,133,1); box-shadow: 9px 7px 52px -6px rgba(133,133,133,1); background-color: rgb(22, 105, 173); } </style> </head> <body> <div class="col-md-3"></div> <div class="col-md-6 well"> <center><h1 id="timer" style="font-size:85px;color:white;font-family: 'Exo 2', sans-serif;">00:00:00s</h1></center> <br /> <div style="text-align:center;"> <button class="btn btn-lg btn-success" onclick="startTimer(this)">START</button> <button class="btn btn-lg btn-danger" onclick="stopTimer()">STOP</button> <button class="btn btn-lg btn-warning" onclick="resetTimer()">RESET</button> </div> </div> <script src="js/script.js"></script> </body> </html>
Creating Script To handle the Stopwatch
In this step, we are going to create a stopwatch function which will be controlled with the help of a button.
/* Created By Source Code Site For More Source Code Visite www.sourcecodessite.com */ var display = document.getElementById('timer'); var secs = 0; var mins = 0; var hrs = 0; var h = ""; var m = ""; var s = ""; var timer; function countTimer(){ secs++; if(secs >= 60){ secs = 0; mins++; if(mins >= 60){ mins = 0; hrs++; } } h = hrs ? hrs > 9 ? hrs : "0" + hrs : "00"; m = mins ? mins > 9 ? mins : "0" + mins : "00"; s = secs > 9 ? secs : "0" + secs; display.innerHTML = h+":"+m+":"+s+"s"; timerDuration(); } function timerDuration(){ if(hrs != 99){ timer = setTimeout(countTimer, 100); } } function startTimer(btn){ btn.setAttribute('disabled', 'disabled'); timerDuration(); } function stopTimer(){ document.getElementsByClassName('btn-success')[0].removeAttribute('disabled'); clearTimeout(timer); } function resetTimer(){ document.getElementsByClassName('btn-success')[0].removeAttribute('disabled'); clearTimeout(timer); display.innerHTML = "00:00:00s"; secs = 0; mins = 0; hrs = 0; h = ""; m = ""; s = ""; }
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.