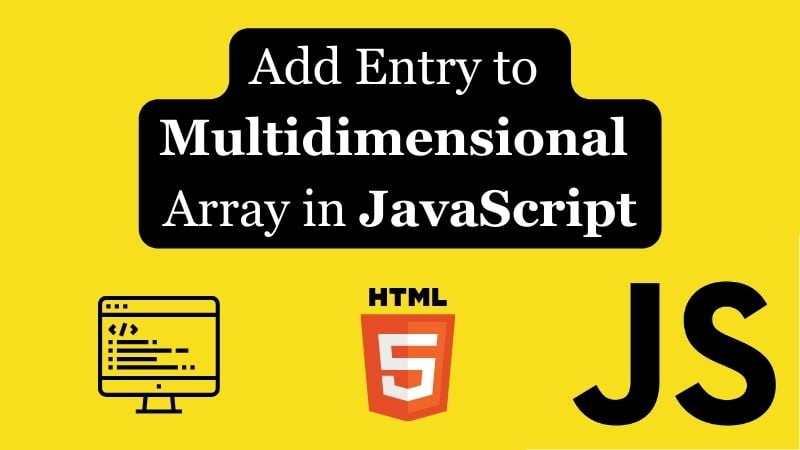
How to Add Entry to Multidimensional Array in Javascript
In JavaScript, a multidimensional array is an array that contains other arrays as its elements. This allows you to create a grid-like structure with rows and columns, where each element in the array can be accessed using multiple indices.
To add an entry to a multidimensional array in JavaScript using HTML, you would typically combine JavaScript code within an HTML file. Let’s break down the process step by step:
Step 1: Set up your HTML structure
Start by creating an HTML file and setting up the necessary structure. For example, you can have a form to trigger the addition of an entry and a container to display the multidimensional array.
<!DOCTYPE html> <html lang="en"> <head> <title>How to Add Entry to Multidimensional Array in Javascript</title> <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/> <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/> <style> .content{ margin-top: 100px; background: white; } </style> </head> <body> <div class="col-md-3 content"></div> <div class="col-md-6 well content"> <h3 class="text-primary">How to Add Entry to Multidimensional Array in Javascript</h3> <hr style="border-top:1px dotted #ccc;"/> <div class="col-md-8"> <pre>[<div id="result"></div>]</pre> </div> <div class="col-md-4"> <div class="form-group"> <label>Firstname</label> <input type="text" id="firstname" class="form-control"/> </div> <div class="form-group"> <label>Lastname</label> <input type="text" id="lastname" class="form-control"/> </div> <div class="form-group"> <label>Address</label> <input type="text" id="address" class="form-control"/> </div> <center><button type="button" onclick="addEntry()" class="btn btn-primary form-control">Add</button></center> </div> </div> </body> <script src="script.js"></script> </html>
In the above HTML code, we have a form with an onclick
attribute set to a JavaScript function called addEntry()
. We also have a <div>
element with an id attribute set to “result” where we will display the multidimensional array.
How to Update HTML list Dynamically using Javascript
Step 2: Write the JavaScript logic
Within the script.js files, write the JavaScript logic to handle adding an entry to the multidimensional array.
var data = [ {firstname: "John", lastname: "Smith", address: "New York"}, {firstname: "Alex", lastname: "Carey", address: "Australia"} ]; displayResult(data); function addEntry(){ let firstname = document.getElementById("firstname"); let lastname = document.getElementById("lastname"); let address = document.getElementById("address"); if(firstname.value == "" || lastname.value == "" || address.value == ""){ alert("Please complete the required field first!"); }else{ var member = { 'firstname': firstname.value, 'lastname': lastname.value, 'address': address.value, }; data.push(member); displayResult(data); } } function displayResult(data){ let html = "" ; for(var i in data){ html += " {firstname:"+ "'"+data[i].firstname+"'"+", "+"lastname:'"+data[i].lastname+"'"+", "+"address:'"+data[i].address+"'"+"}<br />" ; } document.getElementById("result").innerHTML = html; firstname.value = ""; lastname.value = ""; address.value = ""; }
In the JavaScript code above, we first declare the multidimensional array called data with some initial values.
The addEntry()
function is triggered when the button is clicked. Inside this function, we create a new row array and add it to the multidimensional array using the push()
method.
The displayResult()
function is responsible for updating the HTML to reflect the current state of the multidimensional array. It first clears the arrayContainer by setting its innerHTML to an empty string. Then, it iterates over the multidimensional array.
Lastly, we call the displayResult() function initially to show the multidimensional array when the page loads.
If you facing any type of problem with above source code then you can Download the Complete source code in zip Format by clicking the below button. otherwise you can send Comment