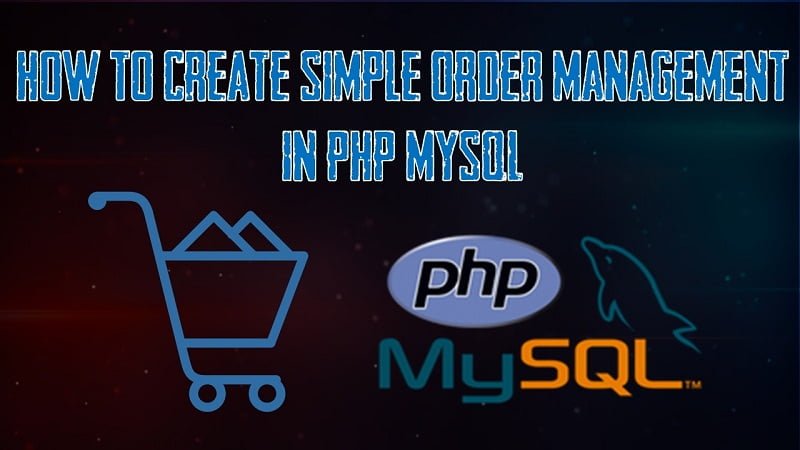
How to create Simple Order Management in PHP MySQL
The Previous Post we have learned How to Create E-commerce Website Script in PHP and in this article we are going to learn simple How to create simple order management system in PHP MySQL. the help of this script you can able to manage products, manage categories and place an order with order history detail. You Can Download The Complete Source Code by clicking Download Source Code in Below.
Getting Bootstrap
Bootstrap can be Download to your needs from their Getting Started page but I would prefer using the CDN option, because it is faster also it is advised to go through and get yourself accustomed with some bootstrap terms, including common classes. This page also some Examples of how to use Bootstrap classes.
1-Creating Database
- Open PHPMyAdmin in your Browser
- Click on Database Tab Display on Topside
- Give the Database name “order”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.8.2 -- https://www.phpmyadmin.net/ -- -- Host: 127.0.0.1 -- Generation Time: Oct 30, 2018 at 11:44 AM -- Server version: 10.1.34-MariaDB -- PHP Version: 5.6.37 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Database: `order` -- -- -------------------------------------------------------- -- -- Table structure for table `category` -- CREATE TABLE `category` ( `categoryid` int(11) NOT NULL, `catname` varchar(30) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `category` -- INSERT INTO `category` (`categoryid`, `catname`) VALUES (13, 'Veg '), (14, 'Non-Veg'), (16, 'Sweats'); -- -------------------------------------------------------- -- -- Table structure for table `product` -- CREATE TABLE `product` ( `productid` int(11) NOT NULL, `categoryid` int(1) NOT NULL, `productname` varchar(30) NOT NULL, `price` double NOT NULL, `photo` varchar(150) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `product` -- INSERT INTO `product` (`productid`, `categoryid`, `productname`, `price`, `photo`) VALUES (23, 13, 'Paneer', 150, 'upload/creamy-matar-paneer-curry.1024x1024-2-225x225_1540894616.jpg'), (24, 13, 'Fried Rice', 120, 'upload/miso-fried-rice-2-225x225_1540894652.jpg'), (25, 14, 'Chicken', 250, 'upload/Easy-Chinese-Chilli-Chicken-Dry-4-225x225_1540894754.jpg'), (27, 16, 'Rasgulla', 25, 'upload/rasgulla-250x250_1540894743.jpeg'); -- -------------------------------------------------------- -- -- Table structure for table `purchase` -- CREATE TABLE `purchase` ( `purchaseid` int(11) NOT NULL, `customer` varchar(50) NOT NULL, `total` double NOT NULL, `date_purchase` datetime NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `purchase` -- INSERT INTO `purchase` (`purchaseid`, `customer`, `total`, `date_purchase`) VALUES (10, 'Raj', 270, '2018-10-30 15:52:43'); -- -------------------------------------------------------- -- -- Table structure for table `purchase_detail` -- CREATE TABLE `purchase_detail` ( `pdid` int(11) NOT NULL, `purchaseid` int(11) NOT NULL, `productid` int(11) NOT NULL, `quantity` int(11) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `purchase_detail` -- INSERT INTO `purchase_detail` (`pdid`, `purchaseid`, `productid`, `quantity`) VALUES (13, 8, 15, 2), (14, 8, 17, 2), (15, 8, 18, 2), (16, 9, 15, 5), (17, 10, 24, 1), (18, 10, 23, 1); -- -- Indexes for dumped tables -- -- -- Indexes for table `category` -- ALTER TABLE `category` ADD PRIMARY KEY (`categoryid`); -- -- Indexes for table `product` -- ALTER TABLE `product` ADD PRIMARY KEY (`productid`); -- -- Indexes for table `purchase` -- ALTER TABLE `purchase` ADD PRIMARY KEY (`purchaseid`); -- -- Indexes for table `purchase_detail` -- ALTER TABLE `purchase_detail` ADD PRIMARY KEY (`pdid`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `category` -- ALTER TABLE `category` MODIFY `categoryid` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=17; -- -- AUTO_INCREMENT for table `product` -- ALTER TABLE `product` MODIFY `productid` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=28; -- -- AUTO_INCREMENT for table `purchase` -- ALTER TABLE `purchase` MODIFY `purchaseid` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=11; -- -- AUTO_INCREMENT for table `purchase_detail` -- ALTER TABLE `purchase_detail` MODIFY `pdid` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=19; COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder.
- Open PHPMyAdmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “order”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find Db file in Downloaded source code Select it.
- Then Click on Go.
Download More Source Code Like
- How to Create Registration with Email Verification in PHP MySQL
- How to Create Multi Step Form in PHP MySQL using Bootstrap
- How To Update Multiple Rows using PHP MySQL
- How To Create Website with admin panel in PHP MySQL
2- Creating Database Connection
After import Database File then next step is creating database connection using PHP copy the below code and save it is as “conn.php”.
<?php $conn = new mysqli("localhost", "root", "", "order"); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } ?>
3 – Create Product & Category Modal Form
in this step, we are going to create product form to add product and category from front-end.
<!-- Add Product --> <div class="modal fade" id="addproduct" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center> <h4 class="modal-title" id="myModalLabel">Add New Product</h4> </center> </div> <div class="modal-body"> <div class="container-fluid"> <form method="POST" action="addproduct.php" enctype="multipart/form-data"> <div class="form-group" style="margin-top:10px;"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Product Name:</label> </div> <div class="col-md-9"> <input type="text" class="form-control" name="pname" required> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Category:</label> </div> <div class="col-md-9"> <select class="form-control" name="category"> <?php $sql="select * from category order by categoryid asc"; $query=$conn->query($sql); while($row=$query->fetch_array()){ ?> <option value=" <?php echo $row['categoryid']; ?>"> <?php echo $row['catname']; ?> </option> <?php } ?> </select> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Price:</label> </div> <div class="col-md-9"> <input type="text" class="form-control" name="price" required> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Photo:</label> </div> <div class="col-md-9"> <input type="file" name="photo"> </div> </div> </div> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> <span class="glyphicon glyphicon-remove"></span> Close </button> <button type="submit" class="btn btn-primary"> <span class="glyphicon glyphicon-floppy-disk"></span> Save </button> </form> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal-dialog --> </div> <!-- Add Category --> <div class="modal fade" id="addcategory" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center> <h4 class="modal-title" id="myModalLabel">Add New Category</h4> </center> </div> <div class="modal-body"> <div class="container-fluid"> <form method="POST" action="addcategory.php" enctype="multipart/form-data"> <div class="form-group" style="margin-top:10px;"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Category Name:</label> </div> <div class="col-md-9"> <input type="text" class="form-control" name="cname" required> </div> </div> </div> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> <span class="glyphicon glyphicon-remove"></span> Close </button> <button type="submit" class="btn btn-primary"> <span class="glyphicon glyphicon-floppy-disk"></span> Save </button> </form> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal-dialog --> </div>
4 – Insert Product Into MySQL Database
in this step we are going to create php script to insert product into mySQL Database.
<?php include "conn.php"; $pname = $_POST["pname"]; $price = $_POST["price"]; $category = $_POST["category"]; $fileinfo = PATHINFO($_FILES["photo"]["name"]); if (empty($fileinfo["filename"])) { $location = ""; } else { $newFilename = $fileinfo["filename"] . "_" . time() . "." . $fileinfo["extension"]; move_uploaded_file($_FILES["photo"]["tmp_name"], "upload/" . $newFilename); $location = "upload/" . $newFilename; } $sql = "insert into product (productname, categoryid, price, photo) values ('$pname', '$category', '$price', '$location')"; $conn->query($sql); header("location:product.php"); ?>
5 – Insert Category Into MySQL Database
in this step we are going to create php script to insert Category into mySQL Database.
<?php include "conn.php"; $cname = $_POST["cname"]; $sql = "insert into category (catname) values ('$cname')"; $conn->query($sql); header("location:category.php"); ?>
6 – manage Products
in this step we are going to show all products in product page and here you can edit and delete any product.
<?php include('includes/header.php'); ?> <body> <?php include('includes/navbar.php'); ?> <div class="container"> <h1 class="page-header text-center">Manage Products</h1> <div class="row"> <div class="col-md-12"> <select id="catList" class="btn btn-default"> <option value="0">All Category</option> <?php $sql="select * from category"; $catquery=$conn->query($sql); while($catrow=$catquery->fetch_array()){ $catid = isset($_GET['category']) ? $_GET['category'] : 0; $selected = ($catid == $catrow['categoryid']) ? " selected" : ""; echo " <option$selected value=".$catrow['categoryid'].">".$catrow['catname']."</option>"; } ?> </select> <a href="#addproduct" data-toggle="modal" class="pull-right btn btn-primary"> <span class="glyphicon glyphicon-plus"></span> Product </a> </div> </div> <div style="margin-top:10px;"> <table class="table table-striped table-bordered"> <thead> <th>Photo</th> <th>Product Name</th> <th>Price</th> <th>Action</th> </thead> <tbody> <?php $where = ""; if(isset($_GET['category'])) { $catid=$_GET['category']; $where = " WHERE product.categoryid = $catid"; } $sql="select * from product left join category on category.categoryid=product.categoryid $where order by product.categoryid asc, productname asc"; $query=$conn->query($sql); while($row=$query->fetch_array()){ ?> <tr> <td> <a href=" <?php if(empty($row['photo'])){echo "upload/noimage.jpg";} else{echo $row['photo'];} ?>"> <img src=" <?php if(empty($row['photo'])){echo "upload/noimage.jpg";} else{echo $row['photo'];} ?>" height="30px" width="40px"> </a> </td> <td> <?php echo $row['productname']; ?> </td> <td>Rs. <?php echo number_format($row['price'], 2); ?> </td> <td> <a href="#editproduct <?php echo $row['productid']; ?>" data-toggle="modal" class="btn btn-success btn-sm"> <span class="glyphicon glyphicon-pencil"></span> Edit </a> - <a href="#deleteproduct <?php echo $row['productid']; ?>" data-toggle="modal" class="btn btn-danger btn-sm"> <span class="glyphicon glyphicon-trash"></span> Delete </a> <?php include('product_modal.php'); ?> </td> </tr> <?php } ?> </tbody> </table> </div> </div> <?php include('modal.php'); ?> <script type="text/javascript"> $(document).ready(function() { $("#catList").on('change', function() { if ($(this).val() == 0) { window.location = 'product.php'; } else { window.location = 'product.php?category=' + $(this).val(); } }); }); </script> </body> </html>
7 – Edit Product Form in Modal
<!-- Edit Product --> <div class="modal fade" id="editproduct <?php echo $row['productid']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center> <h4 class="modal-title" id="myModalLabel">Edit Product</h4> </center> </div> <div class="modal-body"> <div class="container-fluid"> <form method="POST" action="editproduct.php?product= <?php echo $row['productid']; ?>" enctype="multipart/form-data"> <div class="form-group" style="margin-top:10px;"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Product Name:</label> </div> <div class="col-md-9"> <input type="text" class="form-control" value=" <?php echo $row['productname']; ?>" name="pname"> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Category:</label> </div> <div class="col-md-9"> <select class="form-control" name="category"> <option value=" <?php echo $row['categoryid']; ?>"> <?php echo $row['catname']; ?> </option> <?php $sql="select * from category where categoryid != '".$row['categoryid']."'"; $cquery=$conn->query($sql); while($crow=$cquery->fetch_array()){ ?> <option value=" <?php echo $crow['categoryid']; ?>"> <?php echo $crow['catname']; ?> </option> <?php } ?> </select> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Price:</label> </div> <div class="col-md-9"> <input type="text" class="form-control" value=" <?php echo $row['price']; ?>" name="price"> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-3" style="margin-top:7px;"> <label class="control-label">Photo:</label> </div> <div class="col-md-9"> <input type="file" name="photo"> </div> </div> </div> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> <span class="glyphicon glyphicon-remove"></span> Close </button> <button type="submit" class="btn btn-success"> <span class="glyphicon glyphicon-edit"></span> Update </button> </form> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal-dialog --> </div> <!-- Delete Product --> <div class="modal fade" id="deleteproduct <?php echo $row['productid']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center> <h4 class="modal-title" id="myModalLabel">Delete Product</h4> </center> </div> <div class="modal-body"> <h3 class="text-center"> <?php echo $row['productname']; ?> </h3> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> <span class="glyphicon glyphicon-remove"></span> Close </button> <a href="delete_product.php?product= <?php echo $row['productid']; ?>" class="btn btn-danger"> <span class="glyphicon glyphicon-trash"></span> Yes </a> </form> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal-dialog --> </div>
8 – Edit Product PHP Script
<?php include "conn.php"; $id = $_GET["product"]; $pname = $_POST["pname"]; $category = $_POST["category"]; $price = $_POST["price"]; $sql = "select * from product where productid='$id'"; $query = $conn->query($sql); $row = $query->fetch_array(); $fileinfo = PATHINFO($_FILES["photo"]["name"]); if (empty($fileinfo["filename"])) { $location = $row["photo"]; } else { $newFilename = $fileinfo["filename"] . "_" . time() . "." . $fileinfo["extension"]; move_uploaded_file($_FILES["photo"]["tmp_name"], "upload/" . $newFilename); $location = "upload/" . $newFilename; } $sql = "update product set productname='$pname', categoryid='$category', price='$price', photo='$location' where productid='$id'"; $conn->query($sql); header("location:product.php"); ?>
9 – manage Category
in this step we are going to show all products in Category page and here you can edit and delete any product.
<?php include('includes/header.php'); ?> <body> <?php include('includes/navbar.php'); ?> <div class="container"> <h1 class="page-header text-center">Manage Category</h1> <div class="row"> <div class="col-md-12"> <a href="#addcategory" data-toggle="modal" class="pull-right btn btn-primary"> <span class="glyphicon glyphicon-plus"></span> Category </a> </div> </div> <div style="margin-top:10px;"> <table class="table table-striped table-bordered"> <thead> <th>Category Name</th> <th>Action</th> </thead> <tbody> <?php $sql="select * from category order by categoryid asc"; $query=$conn->query($sql); while($row=$query->fetch_array()){ ?> <tr> <td> <?php echo $row['catname']; ?> </td> <td> <a href="#editcategory <?php echo $row['categoryid']; ?>" data-toggle="modal" class="btn btn-success btn-sm"> <span class="glyphicon glyphicon-pencil"></span> Edit </a> - <a href="#deletecategory <?php echo $row['categoryid']; ?>" data-toggle="modal" class="btn btn-danger btn-sm"> <span class="glyphicon glyphicon-trash"></span> Delete </a> <?php include('category_modal.php'); ?> </td> </tr> <?php } ?> </tbody> </table> </div> </div> <?php include('modal.php'); ?> </body></html>
10 – Edit Category Modal Form
<?php include "conn.php"; $id = $_GET["category"]; $cname = $_POST["cname"]; $sql = "update category set catname='$cname' where categoryid='$id'"; $conn->query($sql); header("location:category.php"); ?>
11 – Delete Product PHP Script
<?php include "conn.php"; $id = $_GET["product"]; $sql = "delete from product where productid='$id'"; $conn->query($sql); header("location:product.php"); ?>
12 – Delete Category
<?php include "conn.php"; $id = $_GET["category"]; $sql = "delete from category where categoryid='$id'"; $conn->query($sql); header("location:category.php"); ?>
13 – Show All Products in Index Page Categories Wise
in this step we are going to create index page where show all products category wise.
<?php include('includes/header.php'); ?> <body> <?php include('includes/navbar.php'); ?> <div class="container"> <h1 class="page-header text-center">All Products</h1> <ul class="nav nav-tabs"> <?php $sql="select * from category order by categoryid asc limit 1"; $fquery=$conn->query($sql); $frow=$fquery->fetch_array(); ?> <li class="active"> <a data-toggle="tab" href="# <?php echo $frow['catname'] ?>"> <?php echo $frow['catname'] ?> </a> </li> <?php $sql="select * from category order by categoryid asc"; $nquery=$conn->query($sql); $num=$nquery->num_rows-1; $sql="select * from category order by categoryid asc limit 1, $num"; $query=$conn->query($sql); while($row=$query->fetch_array()){ ?> <li> <a data-toggle="tab" href="# <?php echo $row['catname'] ?>"> <?php echo $row['catname'] ?> </a> </li> <?php } ?> </ul> <div class="tab-content"> <?php $sql="select * from category order by categoryid asc limit 1"; $fquery=$conn->query($sql); $ftrow=$fquery->fetch_array(); ?> <div id=" <?php echo $ftrow['catname']; ?>" class="tab-pane fade in active" style="margin-top:20px;"> <?php $sql="select * from product where categoryid='".$ftrow['categoryid']."'"; $pfquery=$conn->query($sql); $inc=4; while($pfrow=$pfquery->fetch_array()){ $inc = ($inc == 4) ? 1 : $inc+1; if($inc == 1) echo " <div class='row'>"; ?> <div class="col-md-3"> <div class="panel panel-default"> <div class="panel-heading text-center"> <b> <?php echo $pfrow['productname']; ?> </b> </div> <div class="panel-body"> <img src=" <?php if(empty($pfrow['photo'])){echo "upload/noimage.jpg";} else{echo $pfrow['photo'];} ?>" height="225px;" width="100%"> </div> <div class="panel-footer text-center"> Rs. <?php echo number_format($pfrow['price'], 2); ?> </div> </div> </div> <?php if($inc == 4) echo " </div>"; } if($inc == 1) echo " <div class='col-md-3'></div> <div class='col-md-3'></div> <div class='col-md-3'></div> </div>"; if($inc == 2) echo " <div class='col-md-3'></div> <div class='col-md-3'></div> </div>"; if($inc == 3) echo " <div class='col-md-3'></div> </div>"; ?> </div> <?php $sql="select * from category order by categoryid asc"; $tquery=$conn->query($sql); $tnum=$tquery->num_rows-1; $sql="select * from category order by categoryid asc limit 1, $tnum"; $cquery=$conn->query($sql); while($trow=$cquery->fetch_array()){ ?> <div id=" <?php echo $trow['catname']; ?>" class="tab-pane fade" style="margin-top:20px;"> <?php $sql="select * from product where categoryid='".$trow['categoryid']."'"; $pquery=$conn->query($sql); $inc=4; while($prow=$pquery->fetch_array()){ $inc = ($inc == 4) ? 1 : $inc+1; if($inc == 1) echo " <div class='row'>"; ?> <div class="col-md-3"> <div class="panel panel-default"> <div class="panel-heading text-center"> <b> <?php echo $prow['productname']; ?> </b> </div> <div class="panel-body"> <img src=" <?php if($prow['photo']==''){echo "upload/noimage.jpg";} else{echo $prow['photo'];} ?>" height="" width=""> </div> <div class="panel-footer text-center"> ₱ <?php echo number_format($prow['price'], 2); ?> </div> </div> </div> <?php if($inc == 4) echo " </div>"; } if($inc == 1) echo " <div class='col-md-3'></div> <div class='col-md-3'></div> <div class='col-md-3'></div> </div>"; if($inc == 2) echo " <div class='col-md-3'></div> <div class='col-md-3'></div> </div>"; if($inc == 3) echo " <div class='col-md-3'></div> </div>"; ?></div><?php } ?></div> </div></body></html>
14 – Create Order Page For Getting Order
<?php include('includes/header.php'); ?> <body> <?php include('includes/navbar.php'); ?> <div class="container"> <h1 class="page-header text-center">Order Page</h1> <form method="POST" action="purchase.php"> <table class="table table-striped table-bordered"> <thead> <th class="text-center"> <input type="checkbox" id="checkAll"> </th> <th>Category</th> <th>Product Name</th> <th>Price</th> <th>Quantity</th> </thead> <tbody> <?php $sql="select * from product left join category on category.categoryid=product.categoryid order by product.categoryid asc, productname asc"; $query=$conn->query($sql); $iterate=0; while($row=$query->fetch_array()){ ?> <tr> <td class="text-center"> <input type="checkbox" value=" <?php echo $row['productid']; ?>|| <?php echo $iterate; ?>" name="productid[]" style=""> </td> <td> <?php echo $row['catname']; ?> </td> <td> <?php echo $row['productname']; ?> </td> <td class="text-right">Rs. <?php echo number_format($row['price'], 2); ?> </td> <td> <input type="text" class="form-control" name="quantity_ <?php echo $iterate; ?>"> </td> </tr> <?php $iterate++; } ?> </tbody> </table> <div class="row"> <div class="col-md-3"> <input type="text" name="customer" class="form-control" placeholder="Customer Name" required> </div> <div class="col-md-2" style="margin-left:-20px;"> <button type="submit" class="btn btn-primary"> <span class="glyphicon glyphicon-floppy-disk"></span> Save </button> </div> </div> </form> </div> <script type="text/javascript"> $(document).ready(function() { $("#checkAll").click(function() { $('input:checkbox').not(this).prop('checked', this.checked); }); }); </script> </body> </html>
15 – Order Script in PHP
<?php include "conn.php"; if (isset($_POST["productid"])) { $customer = $_POST["customer"]; $sql = "insert into purchase (customer, date_purchase) values ('$customer', NOW())"; $conn->query($sql); $pid = $conn->insert_id; $total = 0; foreach ($_POST["productid"] as $product): $proinfo = explode("||", $product); $productid = $proinfo[0]; $iterate = $proinfo[1]; $sql = "select * from product where productid='$productid'"; $query = $conn->query($sql); $row = $query->fetch_array(); if (isset($_POST["quantity_" . $iterate])) { $subt = $row["price"] * $_POST["quantity_" . $iterate]; $total += $subt; $sql = "insert into purchase_detail (purchaseid, productid, quantity) values ('$pid', '$productid', '" . $_POST["quantity_" . $iterate] . "')"; $conn->query($sql); } endforeach; $sql = "update purchase set total='$total' where purchaseid='$pid'"; $conn->query($sql); header("location:sales.php"); } else { ?> <script> window.alert('Please select a product'); window.location.href='order.php'; </script> <?php } ?>
16 – Show All Order History
<?php include('includes/header.php'); ?> <body> <?php include('includes/navbar.php'); ?> <div class="container"> <h1 class="page-header text-center">Order History</h1> <table class="table table-striped table-bordered"> <thead> <th>Date</th> <th>Customer</th> <th>Total Sales</th> <th>Details</th> </thead> <tbody> <?php $sql="select * from purchase order by purchaseid desc"; $query=$conn->query($sql); while($row=$query->fetch_array()){ ?> <tr> <td> <?php echo date('M d, Y h:i A', strtotime($row['date_purchase'])) ?> </td> <td> <?php echo $row['customer']; ?> </td> <td class="text-right">Rs. <?php echo number_format($row['total'], 2); ?> </td> <td> <a href="#details <?php echo $row['purchaseid']; ?>" data-toggle="modal" class="btn btn-primary btn-sm"> <span class="glyphicon glyphicon-search"></span> View </a> <?php include('sales_modal.php'); ?> </td> </tr> <?php } ?> </tbody> </table> </div> </body></html>
17- Each Order Detail in Modal
<!-- Sales Details --> <div class="modal fade" id="details <?php echo $row['purchaseid']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog modal-lg"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center> <h4 class="modal-title" id="myModalLabel">Order Full Details</h4> </center> </div> <div class="modal-body"> <div class="container-fluid"> <h5>Customer: <b> <?php echo $row['customer']; ?> </b> <span class="pull-right"> <?php echo date('M d, Y h:i A', strtotime($row['date_purchase'])) ?> </span> </h5> <table class="table table-bordered table-striped"> <thead> <th>Product Name</th> <th>Price</th> <th>Purchase Quantity</th> <th>Subtotal</th> </thead> <tbody> <?php $sql="select * from purchase_detail left join product on product.productid=purchase_detail.productid where purchaseid='".$row['purchaseid']."'"; $dquery=$conn->query($sql); while($drow=$dquery->fetch_array()){ ?> <tr> <td> <?php echo $drow['productname']; ?> </td> <td class="text-right">Rs. <?php echo number_format($drow['price'], 2); ?> </td> <td> <?php echo $drow['quantity']; ?> </td> <td class="text-right">Rs. <?php $subt = $drow['price']*$drow['quantity']; echo number_format($subt, 2); ?> </td> </tr> <?php } ?> <tr> <td colspan="3" class="text-right"> <b>TOTAL</b> </td> <td class="text-right">Rs. <?php echo number_format($row['total'], 2); ?> </td> </tr> </tbody> </table> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"> <span class="glyphicon glyphicon-remove"></span> Close </button> </div> </div> <!-- /.modal-content --> </div> <!-- /.modal-dialog --> </div>
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send a Comment.
[aio_button align=”none” animation=”swing” color=”pink” size=”medium” icon=”none” text=”Download Now” relationship=”dofollow” url=”https://www.sourcecodessite.com/wp-content/uploads/2018/10/order.zip”]
thank your sir!