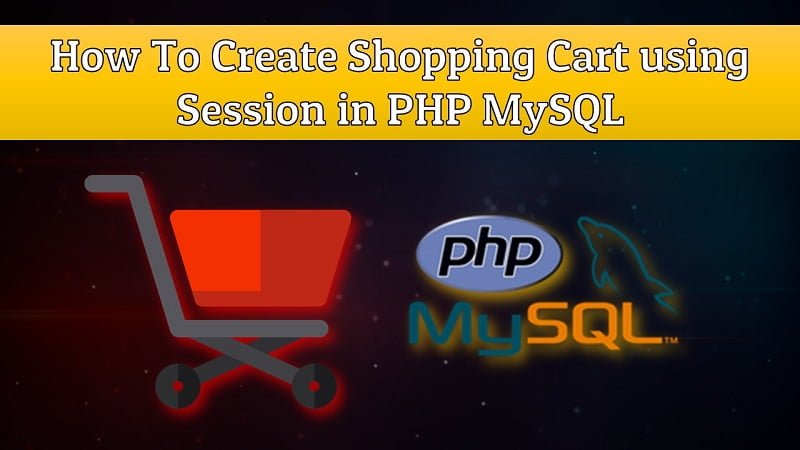
How To Create Shopping Cart using Session in PHP/MySQL
Hi Viewer in this post we are going to teach you How To Create Shopping Cart using Session in PHP/MySQL. I am going to teach how to add products to the shopping cart and how to update and delete product in cart with the help of SESSION in PHP MYSQL.
How To Create Shopping Cart using Session in PHP/MySQL
1-Creating Database
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “shopping”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.8.2 -- https://www.phpmyadmin.net/ -- -- Host: 127.0.0.1 -- Generation Time: Oct 11, 2018 at 10:15 PM -- Server version: 10.1.34-MariaDB -- PHP Version: 5.6.37 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Database: `shopping` -- -- -------------------------------------------------------- -- -- Table structure for table `products` -- CREATE TABLE `products` ( `id` int(11) NOT NULL, `name` varchar(200) NOT NULL, `price` double NOT NULL, `photo` varchar(150) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `products` -- INSERT INTO `products` (`id`, `name`, `price`, `photo`) VALUES (1, 'Headphone', 599, 'images/1.png'), (2, 'Mobile', 12000, 'images/2.jpg'), (3, 'Keyboard', 369, 'images/3.jpg'), (4, 'Mouse', 150, 'images/4.jpg'), (5, 'Laptop Bag', 698, 'images/5.jpg'), (6, 'Laptop', 22050, 'images/6.png'); -- -- Indexes for dumped tables -- -- -- Indexes for table `products` -- ALTER TABLE `products` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `products` -- ALTER TABLE `products` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=7; COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder.
- Open PHPMyAdmin in your Browser
- Click on Database Tab Display on Topside
- Give the Database name “shopping”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find db folder in the Downloaded source code where find shopping.sql file then Select it.
- Then Click on Go.
Download More Source Code Like
- How to Create Registration with Email Verification in PHP MySQL
- How to Create Multi Step Form in PHP MySQL using Bootstrap
- How To Update Multiple Rows using PHP MySQL
- How To Create Website with admin panel in PHP MySQL
2 – Creating Index Page Where Show Products
in this step we are going to show you how to create index page and how to show products from mysql database using php. you can copy the below code and save it as index.php in your project folder.
<?php session_start(); //initialize cart if not set or is unset if(!isset($_SESSION['cart'])){ $_SESSION['cart'] = array(); } //unset qunatity unset($_SESSION['qty_array']); ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>How TO Create Shopping Cart using Session in PHP/MySQL</title> <link rel="stylesheet" type="text/css" href="bootstrap/css/bootstrap.min.css"> <style> body{ margin-top:25px; } .product_image{ height:200px; } .product_name{ height:80px; padding-left:20px; padding-right:20px; } .product_footer{ padding-left:20px; padding-right:20px; } </style> </head> <body> <div class="container"> <nav class="navbar navbar-default"> <div class="container-fluid"> <div class="navbar-header"> <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1" aria-expanded="false"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="#">How TO Create Shopping Cart using Session in PHP/MySQL</a> </div> <div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1"> <ul class="nav navbar-nav"> <!-- left nav here --> </ul> <ul class="nav navbar-nav navbar-right"> <li><a href="view_cart.php"><span class="badge"><?php echo count($_SESSION['cart']); ?></span> Shopping Cart <span class="glyphicon glyphicon-shopping-cart"></span></a></li> </ul> </div> </div> </nav> <?php //info message if(isset($_SESSION['message'])){ ?> <div class="row"> <div class="col-sm-6 col-sm-offset-6"> <div class="alert alert-info text-center"> <?php echo $_SESSION['message']; ?> </div> </div> </div> <?php unset($_SESSION['message']); } //end info message //fetch our products //connection $conn = new mysqli('localhost', 'root', '', 'shopping'); $sql = "SELECT * FROM products"; $query = $conn->query($sql); $inc = 4; while($row = $query->fetch_assoc()){ $inc = ($inc == 4) ? 1 : $inc + 1; if($inc == 1) echo "<div class='row text-center'>"; ?> <div class="col-sm-3"> <div class="panel panel-default"> <div class="panel-body"> <div class="row product_image"> <img src="<?php echo $row['photo'] ?>" width="80%" height="auto"> </div> <div class="row product_name"> <h4><?php echo $row['name']; ?></h4> </div> <div class="row product_footer"> <p class="pull-left"><b><?php echo $row['price']; ?></b></p> <span class="pull-right"><a href="add_cart.php?id=<?php echo $row['id']; ?>" class="btn btn-primary btn-sm"><span class="glyphicon glyphicon-plus"></span> Cart</a></span> </div> </div> </div> </div> <?php } if($inc == 1) echo "<div></div><div></div><div></div></div>"; if($inc == 2) echo "<div></div><div></div></div>"; if($inc == 3) echo "<div></div></div>"; //end product row ?> </div> </body> </html>
3 – Create Add To Cart Script in PHP
in this step we aregoing to create add to cart script using php . this scripts perform add to cart button through php session. you can add a file and copy the below source code and save it as add_cart.php.
<?php session_start(); if(!in_array($_GET['id'], $_SESSION['cart'])){ array_push($_SESSION['cart'], $_GET['id']); $_SESSION['message'] = 'Product added to cart'; } else{ $_SESSION['message'] = 'Product already in cart'; } header('location: index.php'); ?>
4 – Creating a View Shopping Cart Page
in this step we are going to create view cart page. after adding product in cart then this page show how many products uin your cart and user can manage shopping cart easily. copy the bleow code and save it as view_cart.php.
<?php session_start(); ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>How To Create Shopping Cart using Session in PHP/MySQL</title> <link rel="stylesheet" type="text/css" href="bootstrap/css/bootstrap.min.css"> <style> body{ margin-top:25px; } </style> </head> <body> <div class="container"> <nav class="navbar navbar-default"> <div class="container-fluid"> <div class="navbar-header"> <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1" aria-expanded="false"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="#">How TO Create Shopping Cart using Session in PHP/MySQL</a> </div> <div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1"> <ul class="nav navbar-nav"> <!-- left nav here --> </ul> <ul class="nav navbar-nav navbar-right"> <li class="active"><a href="view_cart.php"><span class="badge"><?php echo count($_SESSION['cart']); ?></span> Cart <span class="glyphicon glyphicon-shopping-cart"></span></a></li> </ul> </div> </div> </nav> <h1 class="page-header text-center">Shopping Cart Details</h1> <div class="row"> <div class="col-sm-8 col-sm-offset-2"> <?php if(isset($_SESSION['message'])){ ?> <div class="alert alert-info text-center"> <?php echo $_SESSION['message']; ?> </div> <?php unset($_SESSION['message']); } ?> <form method="POST" action="save_cart.php"> <table class="table table-bordered table-striped"> <thead> <th>Name</th> <th>Price</th> <th>Quantity</th> <th>Subtotal</th> <th>Action</th> </thead> <tbody> <?php //initialize total $total = 0; if(!empty($_SESSION['cart'])){ //connection $conn = new mysqli('localhost', 'root', '', 'shopping'); //create array of initail qty which is 1 $index = 0; if(!isset($_SESSION['qty_array'])){ $_SESSION['qty_array'] = array_fill(0, count($_SESSION['cart']), 1); } $sql = "SELECT * FROM products WHERE id IN (".implode(',',$_SESSION['cart']).")"; $query = $conn->query($sql); while($row = $query->fetch_assoc()){ ?> <tr> <td><?php echo $row['name']; ?></td> <td><?php echo number_format($row['price'], 2); ?></td> <input type="hidden" name="indexes[]" value="<?php echo $index; ?>"> <td><input type="text" class="form-control" value="<?php echo $_SESSION['qty_array'][$index]; ?>" name="qty_<?php echo $index; ?>"></td> <td><?php echo number_format($_SESSION['qty_array'][$index]*$row['price'], 2); ?></td> <?php $total += $_SESSION['qty_array'][$index]*$row['price']; ?> <td> <a href="delete_item.php?id=<?php echo $row['id']; ?>&index=<?php echo $index; ?>" class="btn btn-danger btn-sm"><span class="glyphicon glyphicon-trash"></span></a> </td> </tr> <?php $index ++; } } else{ ?> <tr> <td colspan="4" class="text-center">No Item in Cart</td> </tr> <?php } ?> <tr> <td colspan="4" align="right"><b>Total</b></td> <td><b><?php echo number_format($total, 2); ?></b></td> </tr> </tbody> </table> <a href="index.php" class="btn btn-primary"><span class="glyphicon glyphicon-arrow-left"></span> Back to Shopping</a> <button type="submit" class="btn btn-success" name="save">Update Cart</button> <a href="clear_cart.php" class="btn btn-danger"><span class="glyphicon glyphicon-trash"></span> Clear Cart</a> </form> </div> </div> </div> </body> </html>
5 – Delete Products From Cart using PHP
in this step we are going to create a script to delete products easily from shopping cart. copy the below code and save it as delete_item.php.
<?php session_start(); $key = array_search($_GET['id'], $_SESSION['cart']); unset($_SESSION['cart'][$key]); unset($_SESSION['qty_array'][$_GET['index']]); $_SESSION['qty_array'] = array_values($_SESSION['qty_array']); $_SESSION['message'] = "Product deleted from cart"; header('location: view_cart.php'); ?>
6 – Update Product Quantity in Cart using PHP
int his step we are going to create update quantity script to performs users can easily imcrease product quantity in cart page product price calculate automatically. copy the below code and save it as save_cart.php.
<?php session_start(); if(isset($_POST['save'])){ foreach($_POST['indexes'] as $key){ $_SESSION['qty_array'][$key] = $_POST['qty_'.$key]; } $_SESSION['message'] = 'Cart updated successfully'; header('location: view_cart.php'); } ?>
7 – Delete total Product in a Cart using PHP
in this step we are going to create delete total product in a cart script using php. you can copy rhe below source source code and save it as clear_cart.php.
<?php session_start(); unset($_SESSION['cart']); $_SESSION['message'] = 'Cart cleared successfully'; header('location: index.php'); ?>
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.