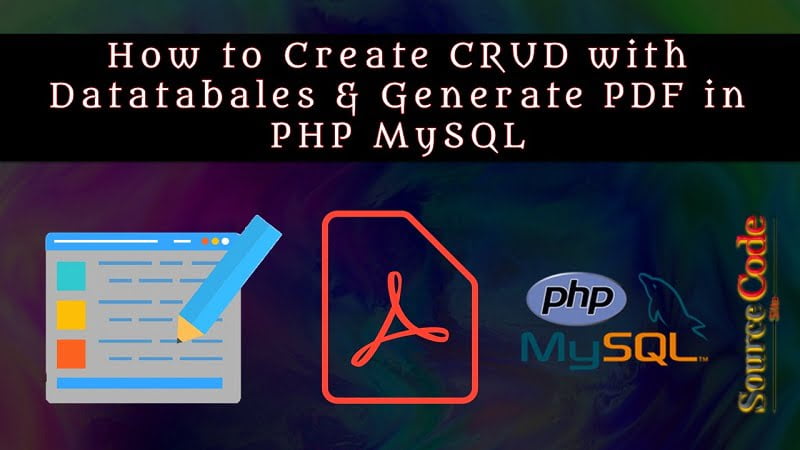
How to Create CRUD with Datatabales & Generate PDF in PHP MySQL
In the Previous article, we have already learned How to use bootstrap tables to Display data from MySQL using PHP and in this article, we are going to learn how to create CRUD with Databases & Generate PDF in PHP MySQL. and generate PDF with the help of TCPDF. as we know Bootstrap is a very popular open source project that provides us more option in web design. Bootstrap is a CSS based framework that can easily be integrated with minimum customization.
Getting Bootstrap
Bootstrap can be Download to your needs from their Getting Started page but i would prefer using the CDN option, because it is faster also it is advised to go through and get yourself accustomed with some bootstrap terms, including common classes. This page also some Examples of how to use Bootstrap classes.
1-Creating Database
- Open PHPMyAdmin in your Browser
- Click on Database Tab Display on Topside
- Give the Database name “crud”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.8.2 -- https://www.phpmyadmin.net/ -- -- Host: 127.0.0.1 -- Generation Time: Oct 28, 2018 at 02:28 PM -- Server version: 10.1.34-MariaDB -- PHP Version: 5.6.37 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET AUTOCOMMIT = 0; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Database: `crud` -- -- -------------------------------------------------------- -- -- Table structure for table `members` -- CREATE TABLE `members` ( `id` int(11) NOT NULL, `firstname` varchar(30) NOT NULL, `lastname` varchar(30) NOT NULL, `address` text NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; -- -- Dumping data for table `members` -- INSERT INTO `members` (`id`, `firstname`, `lastname`, `address`) VALUES (38, 'Deepak', 'Raj', 'Gomati Nagar, 79, 79'), (39, 'Mohit', 'Sharma ', 'Lucknow'), (40, 'Sujeet', 'Sharma', 'India'), (41, 'Alkesh', 'Singh', 'America '), (42, 'Priyanshu', 'Raj', 'India'), (43, 'Deepu', 'Kumar', 'India'), (44, 'Rajan', 'Kumar', 'India'); -- -- Indexes for dumped tables -- -- -- Indexes for table `members` -- ALTER TABLE `members` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `members` -- ALTER TABLE `members` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=45; COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder.
- Open PHPMyAdmin in your Browser
- Click on Database Tab Display on Topside
- Give the Database name “crud”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find Db folder in Downloaded source code Select it.
- Then Click on Go.
Download More Source Code Like
- How to Create Registration with Email Verification in PHP MySQL
- How to Create Multi Step Form in PHP MySQL using Bootstrap
- How To Update Multiple Rows using PHP MySQL
- How To Create Website with admin panel in PHP MySQL
2- Creating Database Connection
After import Database File then next step is creating a database connection using php copy the below code and save it is as “connection.php”.
<?php $conn = new mysqli('localhost', 'root', '', 'crud'); if($conn->connect_error){ die("Connection failed: " . $conn->connect_error); } ?>
3 – Creating Index Page
In this step, we are going to create index page where create datatable and show data from MySQL Database with help of PHP.
<?php session_start(); ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>How Create CRUD with Databales & Generate PDF in PHP MySQL</title> <link rel="stylesheet" type="text/css" href="bootstrap/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="datatable/dataTable.bootstrap.min.css"> <style> .height10{ height:10px; } .mtop10{ margin-top:10px; } .modal-label{ position:relative; top:7px } </style> </head> <body> <div class="container"> <h1 class="page-header text-center">CRUD with Databales & Generate PDF in PHP MySQL</h1> <div class="row"> <div class="col-sm-8 col-sm-offset-2"> <div class="row"> <?php if(isset($_SESSION['error'])){ echo " <div class='alert alert-danger text-center'> <button class='close'>×</button> ".$_SESSION['error']." </div> "; unset($_SESSION['error']); } if(isset($_SESSION['success'])){ echo " <div class='alert alert-success text-center'> <button class='close'>×</button> ".$_SESSION['success']." </div> "; unset($_SESSION['success']); } ?> </div> <div class="row"> <a href="#addnew" data-toggle="modal" class="btn btn-primary"><span class="glyphicon glyphicon-plus"></span> New</a> <a href="print_pdf.php" class="btn btn-success pull-right"><span class="glyphicon glyphicon-print"></span> PDF</a> </div> <div class="height10"> </div> <div class="row"> <table id="myTable" class="table table-bordered table-striped"> <thead> <th>ID</th> <th>Firstname</th> <th>Lastname</th> <th>Address</th> <th>Action</th> </thead> <tbody> <?php include_once('connection.php'); $sql = "SELECT * FROM members"; //use for MySQLi-OOP $query = $conn->query($sql); while($row = $query->fetch_assoc()){ echo "<tr> <td>".$row['id']."</td> <td>".$row['firstname']."</td> <td>".$row['lastname']."</td> <td>".$row['address']."</td> <td> <a href='#edit_".$row['id']."' class='btn btn-success btn-sm' data-toggle='modal'><span class='glyphicon glyphicon-edit'></span> Edit</a> <a href='#delete_".$row['id']."' class='btn btn-danger btn-sm' data-toggle='modal'><span class='glyphicon glyphicon-trash'></span> Delete</a> </td> </tr>"; include('edit_delete_modal.php'); } ?> </tbody> </table> </div> </div> </div> </div> <?php include('add_modal.php') ?> <script src="jquery/jquery.min.js"></script> <script src="bootstrap/js/bootstrap.min.js"></script> <script src="datatable/jquery.dataTables.min.js"></script> <script src="datatable/dataTable.bootstrap.min.js"></script> <!-- generate datatable on our table --> <script> $(document).ready(function(){ //inialize datatable $('#myTable').DataTable(); //hide alert $(document).on('click', '.close', function(){ $('.alert').hide(); }) }); </script> </body> </html>
4 – Creating Add Modal Form
in this step, we are going to create modal form to add data into database.
<!-- Add New --> <div class="modal fade" id="addnew" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center><h4 class="modal-title" id="myModalLabel">Add New</h4></center> </div> <div class="modal-body"> <div class="container-fluid"> <form method="POST" action="add.php"> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Firstname:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="firstname" required> </div> </div> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Lastname:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="lastname" required> </div> </div> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Address:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="address" required> </div> </div> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Cancel</button> <button type="submit" name="add" class="btn btn-primary"><span class="glyphicon glyphicon-floppy-disk"></span> Save</a> </form> </div> </div> </div> </div>
5 – Add Data Into database
In this step we are going to add data into database using PHP.
<?php session_start(); include_once('connection.php'); if(isset($_POST['add'])){ $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $address = $_POST['address']; $sql = "INSERT INTO members (firstname, lastname, address) VALUES ('$firstname', '$lastname', '$address')"; if($conn->query($sql)){ $_SESSION['success'] = 'Record added successfully'; } else{ $_SESSION['error'] = 'Something went wrong while adding'; } } else{ $_SESSION['error'] = 'Fill up add form first'; } header('location: index.php'); ?>
6 – Edit & Delete Modal Form
Next Step is creating edit modal form and delete confirmation modal.
<!-- Edit --> <div class="modal fade" id="edit_<?php echo $row['id']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center><h4 class="modal-title" id="myModalLabel">Edit Member</h4></center> </div> <div class="modal-body"> <div class="container-fluid"> <form method="POST" action="edit.php"> <input type="hidden" class="form-control" name="id" value="<?php echo $row['id']; ?>"> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Firstname:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="firstname" value="<?php echo $row['firstname']; ?>"> </div> </div> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Lastname:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="lastname" value="<?php echo $row['lastname']; ?>"> </div> </div> <div class="row form-group"> <div class="col-sm-2"> <label class="control-label modal-label">Address:</label> </div> <div class="col-sm-10"> <input type="text" class="form-control" name="address" value="<?php echo $row['address']; ?>"> </div> </div> </div> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Cancel</button> <button type="submit" name="edit" class="btn btn-success"><span class="glyphicon glyphicon-check"></span> Update</a> </form> </div> </div> </div> </div> <!-- Delete --> <div class="modal fade" id="delete_<?php echo $row['id']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <center><h4 class="modal-title" id="myModalLabel">Delete Member</h4></center> </div> <div class="modal-body"> <p class="text-center">Are you sure you want to Delete</p> <h2 class="text-center"><?php echo $row['firstname'].' '.$row['lastname']; ?></h2> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Cancel</button> <a href="delete.php?id=<?php echo $row['id']; ?>" class="btn btn-danger"><span class="glyphicon glyphicon-trash"></span> Yes</a> </div> </div> </div> </div>
7 – Edit data using PHP
in this step, we are edit inserted data in database using php.
<?php session_start(); include_once('connection.php'); if(isset($_POST['edit'])){ $id = $_POST['id']; $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $address = $_POST['address']; $sql = "UPDATE members SET firstname = '$firstname', lastname = '$lastname', address = '$address' WHERE id = '$id'"; if($conn->query($sql)){ $_SESSION['success'] = 'Member updated successfully'; } else{ $_SESSION['error'] = 'Something went wrong in updating member'; } } else{ $_SESSION['error'] = 'Select member to edit first'; } header('location: index.php'); ?>
8 – Delete added Data
in this step we are going to delete data from frontend using php.
<?php session_start(); include_once('connection.php'); if(isset($_GET['id'])){ $sql = "DELETE FROM members WHERE id = '".$_GET['id']."'"; if($conn->query($sql)){ $_SESSION['success'] = 'Member deleted successfully'; } else{ $_SESSION['error'] = 'Something went wrong in deleting member'; } } else{ $_SESSION['error'] = 'Select member to delete first'; } header('location: index.php'); ?>
9 – Final Step Generate PDF
in this step, we are going to create pdf generator with the help of TCPDF library in PHP.
<?php function generateRow(){ $contents = ''; include_once('connection.php'); $sql = "SELECT * FROM members"; $query = $conn->query($sql); while($row = $query->fetch_assoc()){ $contents .= " <tr> <td>".$row['id']."</td> <td>".$row['firstname']."</td> <td>".$row['lastname']."</td> <td>".$row['address']."</td> </tr> "; } return $contents; } require_once('tcpdf/tcpdf.php'); $pdf = new TCPDF('P', PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false); $pdf->SetCreator(PDF_CREATOR); $pdf->SetTitle("Generated PDF using TCPDF"); $pdf->SetHeaderData('', '', PDF_HEADER_TITLE, PDF_HEADER_STRING); $pdf->setHeaderFont(Array(PDF_FONT_NAME_MAIN, '', PDF_FONT_SIZE_MAIN)); $pdf->setFooterFont(Array(PDF_FONT_NAME_DATA, '', PDF_FONT_SIZE_DATA)); $pdf->SetDefaultMonospacedFont('helvetica'); $pdf->SetFooterMargin(PDF_MARGIN_FOOTER); $pdf->SetMargins(PDF_MARGIN_LEFT, '10', PDF_MARGIN_RIGHT); $pdf->setPrintHeader(false); $pdf->setPrintFooter(false); $pdf->SetAutoPageBreak(TRUE, 10); $pdf->SetFont('helvetica', '', 11); $pdf->AddPage(); $content = ''; $content .= ' <h2 align="center">All Record Generated in PDF</h2> <h4>Members Table</h4> <table border="1" cellspacing="0" cellpadding="3"> <tr> <th width="5%">ID</th> <th width="20%">Firstname</th> <th width="20%">Lastname</th> <th width="55%">Address</th> </tr> '; $content .= generateRow(); $content .= '</table>'; $pdf->writeHTML($content); $pdf->Output('members.pdf', 'I'); ?>
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.
nice project